Note
Go to the end to download the full example code.
Huge Ensembles (HENS) Checkpoints#
Basic multi-checkpoint Huge Ensembles (HENS) inference workflow.
This example provides a basic example to load the Huge Ensemble checkpoints to perform ensemble inference. This notebook aims to demonstrate the foundations of running a multi-checkpoint workflow from Earth2Studio components. For more details about HENS, see:
Warning
We encourage users to familiarize themselves with the license restrictions of this model’s checkpoints.
For the complete HENS workflow, we encourage users to have a look at the HENS recipe which provides a end-to-end solution to leverage HENS for downstream analysis such as tropical cyclone tracking:
coming soon
In this example you will learn:
How to load the HENS checkpoints with a custom model package
How to load the HENS perturbation method
How to create a simple ensemble inference loop
How to visualize results
Set Up#
First, import the necessary modules and set up our environment and load the required modules. HENS has checkpoints conveniently stored on HuggingFace that we will use. Rather than loading the default checkpoint from the original SFNO paper, create a model package that points to the specific HENS checkpoint we want to use instead.
This example also needs the following:
Prognostic Base Model: Use SFNO model architecture
earth2studio.models.px.SFNO
.Datasource: Pull data from the GFS data api
earth2studio.data.GFS
.Perturbation Method: HENS uses a novel perturbation method
earth2studio.perturbation.HemisphericCentredBredVector
.Seeding Perturbation Method: Perturbation method to seed the Bred Vector
earth2studio.perturbation.CorrelatedSphericalGaussian
.
import os
os.makedirs("outputs", exist_ok=True)
from dotenv import load_dotenv
load_dotenv() # TODO: make common example prep function
from earth2studio.data import GFS
from earth2studio.io import ZarrBackend
from earth2studio.models.auto import Package
from earth2studio.models.px import SFNO
from earth2studio.perturbation import (
CorrelatedSphericalGaussian,
HemisphericCentredBredVector,
)
from earth2studio.run import ensemble
# Set up two model packages for each checkpoint
# Note the modification of the cache location to avoid overwriting
model_package_1 = Package(
"hf://datasets/maheshankur10/hens/earth2mip_prod_registry/sfno_linear_74chq_sc2_layers8_edim620_wstgl2-epoch70_seed102",
cache_options={
"cache_storage": Package.default_cache("hens_1"),
"same_names": True,
},
)
model_package_2 = Package(
"hf://datasets/maheshankur10/hens/earth2mip_prod_registry/sfno_linear_74chq_sc2_layers8_edim620_wstgl2-epoch70_seed103",
cache_options={
"cache_storage": Package.default_cache("hens_2"),
"same_names": True,
},
)
# Create the data source
data = GFS()
Execute the Workflow#
Next we execute the ensemble workflow for each model but loop through each checkpoint. Note that the models themselves have not been loaded into memory yet, this will be done one at a time to minimize the memory footprint of inference on a GPU. Before the ensemble workflow can get executed the following set up is needed:
Initialize the SFNO model from checkpoint
Initialize the perturbation method with the prognostic model
Initialize the IO zarr store for this model
If multiple GPUs are being used, one could parallelize inference using different checkpoints on each card.
import gc
from datetime import datetime, timedelta
import numpy as np
import torch
start_date = datetime(2024, 1, 1)
nsteps = 4
nensemble = 2
for i, package in enumerate([model_package_1, model_package_2]):
# Load SFNO model from package
# HENS checkpoints use different inputs than default SFNO (inclusion of d2m)
# Can find this in the config.json, the load_model function in SFNO handles this
model = SFNO.load_model(package)
# Perturbation method
# Here we will simplify the process that's in the original paper for conciseness
noise_amplification = torch.zeros(model.input_coords()["variable"].shape[0])
noise_amplification[40] = 1.0 # z500
noise_amplification = noise_amplification.reshape(1, 1, 1, -1, 1, 1)
seed_perturbation = CorrelatedSphericalGaussian(noise_amplitude=noise_amplification)
perturbation = HemisphericCentredBredVector(
model, data, seed_perturbation, noise_amplitude=noise_amplification
)
# IO object
io = ZarrBackend(
file_name=f"outputs/11_hens_{i}.zarr",
chunks={"ensemble": 1, "time": 1, "lead_time": 1},
backend_kwargs={"overwrite": True},
)
io = ensemble(
["2024-01-01"],
nsteps,
nensemble,
model,
data,
io,
perturbation,
batch_size=1,
output_coords={"variable": np.array(["u10m", "v10m"])},
)
print(io.root.tree())
# Do some manual clean up to free up VRAM
del model
del perturbation
gc.collect()
torch.cuda.empty_cache()
Downloading config.json: 0%| | 0.00/22.4k [00:00<?, ?B/s]
Downloading config.json: 100%|██████████| 22.4k/22.4k [00:00<00:00, 121kB/s]
Downloading config.json: 100%|██████████| 22.4k/22.4k [00:00<00:00, 121kB/s]
Downloading orography.nc: 0%| | 0.00/2.50M [00:00<?, ?B/s]
Downloading orography.nc: 100%|██████████| 2.50M/2.50M [00:00<00:00, 5.75MB/s]
Downloading orography.nc: 100%|██████████| 2.50M/2.50M [00:00<00:00, 5.68MB/s]
Downloading land_mask.nc: 0%| | 0.00/748k [00:00<?, ?B/s]
Downloading land_mask.nc: 100%|██████████| 748k/748k [00:00<00:00, 5.77MB/s]
Downloading land_mask.nc: 100%|██████████| 748k/748k [00:00<00:00, 5.66MB/s]
Downloading global_means.npy: 0%| | 0.00/720 [00:00<?, ?B/s]
Downloading global_means.npy: 100%|██████████| 720/720 [00:00<00:00, 5.33kB/s]
Downloading global_means.npy: 100%|██████████| 720/720 [00:00<00:00, 5.28kB/s]
Downloading global_stds.npy: 0%| | 0.00/720 [00:00<?, ?B/s]
Downloading global_stds.npy: 100%|██████████| 720/720 [00:00<00:00, 5.42kB/s]
Downloading global_stds.npy: 100%|██████████| 720/720 [00:00<00:00, 5.36kB/s]
Downloading best_ckpt_mp0.tar: 0%| | 0.00/8.31G [00:00<?, ?B/s]
Downloading best_ckpt_mp0.tar: 0%| | 10.0M/8.31G [00:00<04:20, 34.2MB/s]
Downloading best_ckpt_mp0.tar: 0%| | 30.0M/8.31G [00:00<01:52, 79.3MB/s]
Downloading best_ckpt_mp0.tar: 1%| | 50.0M/8.31G [00:00<01:19, 112MB/s]
Downloading best_ckpt_mp0.tar: 1%| | 70.0M/8.31G [00:00<01:05, 134MB/s]
Downloading best_ckpt_mp0.tar: 1%| | 90.0M/8.31G [00:00<01:00, 146MB/s]
Downloading best_ckpt_mp0.tar: 1%|▏ | 110M/8.31G [00:00<00:56, 156MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 130M/8.31G [00:01<00:53, 165MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 150M/8.31G [00:01<00:51, 169MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 170M/8.31G [00:01<00:51, 170MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 190M/8.31G [00:01<00:50, 173MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 210M/8.31G [00:01<00:50, 174MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 230M/8.31G [00:01<00:49, 175MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 250M/8.31G [00:01<00:49, 177MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 270M/8.31G [00:01<00:49, 175MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 290M/8.31G [00:01<00:48, 177MB/s]
Downloading best_ckpt_mp0.tar: 4%|▎ | 310M/8.31G [00:02<00:48, 177MB/s]
Downloading best_ckpt_mp0.tar: 4%|▍ | 330M/8.31G [00:02<00:48, 177MB/s]
Downloading best_ckpt_mp0.tar: 4%|▍ | 350M/8.31G [00:02<00:48, 177MB/s]
Downloading best_ckpt_mp0.tar: 4%|▍ | 370M/8.31G [00:02<00:48, 177MB/s]
Downloading best_ckpt_mp0.tar: 5%|▍ | 390M/8.31G [00:02<00:48, 176MB/s]
Downloading best_ckpt_mp0.tar: 5%|▍ | 410M/8.31G [00:02<00:47, 178MB/s]
Downloading best_ckpt_mp0.tar: 5%|▌ | 430M/8.31G [00:02<00:47, 179MB/s]
Downloading best_ckpt_mp0.tar: 5%|▌ | 450M/8.31G [00:02<00:47, 179MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 470M/8.31G [00:03<00:47, 179MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 490M/8.31G [00:03<00:46, 180MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 510M/8.31G [00:03<00:47, 175MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 530M/8.31G [00:03<00:48, 173MB/s]
Downloading best_ckpt_mp0.tar: 6%|▋ | 550M/8.31G [00:03<00:48, 171MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 570M/8.31G [00:03<00:47, 175MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 590M/8.31G [00:03<00:46, 178MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 610M/8.31G [00:03<00:46, 177MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 630M/8.31G [00:04<00:47, 174MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 650M/8.31G [00:04<00:48, 171MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 670M/8.31G [00:04<00:47, 171MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 690M/8.31G [00:04<00:48, 171MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 710M/8.31G [00:04<00:47, 171MB/s]
Downloading best_ckpt_mp0.tar: 9%|▊ | 730M/8.31G [00:04<00:48, 170MB/s]
Downloading best_ckpt_mp0.tar: 9%|▉ | 750M/8.31G [00:04<00:48, 169MB/s]
Downloading best_ckpt_mp0.tar: 9%|▉ | 770M/8.31G [00:04<00:46, 173MB/s]
Downloading best_ckpt_mp0.tar: 9%|▉ | 790M/8.31G [00:04<00:44, 181MB/s]
Downloading best_ckpt_mp0.tar: 10%|▉ | 810M/8.31G [00:05<00:43, 184MB/s]
Downloading best_ckpt_mp0.tar: 10%|▉ | 830M/8.31G [00:05<00:44, 183MB/s]
Downloading best_ckpt_mp0.tar: 10%|▉ | 850M/8.31G [00:05<00:45, 178MB/s]
Downloading best_ckpt_mp0.tar: 10%|█ | 870M/8.31G [00:05<00:45, 176MB/s]
Downloading best_ckpt_mp0.tar: 10%|█ | 890M/8.31G [00:05<00:45, 174MB/s]
Downloading best_ckpt_mp0.tar: 11%|█ | 910M/8.31G [00:05<00:45, 173MB/s]
Downloading best_ckpt_mp0.tar: 11%|█ | 930M/8.31G [00:05<00:46, 172MB/s]
Downloading best_ckpt_mp0.tar: 11%|█ | 950M/8.31G [00:05<00:44, 176MB/s]
Downloading best_ckpt_mp0.tar: 11%|█▏ | 970M/8.31G [00:06<00:43, 180MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 990M/8.31G [00:06<00:43, 181MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 0.99G/8.31G [00:06<00:44, 175MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 1.01G/8.31G [00:06<00:43, 180MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 1.03G/8.31G [00:06<00:42, 183MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.04G/8.31G [00:06<00:42, 185MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.06G/8.31G [00:06<00:41, 186MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.08G/8.31G [00:06<00:42, 184MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.10G/8.31G [00:06<00:42, 180MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▎ | 1.12G/8.31G [00:07<00:43, 178MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.14G/8.31G [00:07<00:44, 174MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.16G/8.31G [00:07<00:44, 172MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.18G/8.31G [00:07<00:45, 170MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.20G/8.31G [00:07<00:44, 170MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▍ | 1.22G/8.31G [00:07<00:45, 168MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▍ | 1.24G/8.31G [00:07<00:43, 173MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▌ | 1.26G/8.31G [00:07<00:42, 177MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▌ | 1.28G/8.31G [00:08<00:41, 180MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▌ | 1.30G/8.31G [00:08<00:41, 180MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▌ | 1.32G/8.31G [00:08<00:41, 180MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▌ | 1.34G/8.31G [00:08<00:42, 177MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▋ | 1.36G/8.31G [00:08<00:42, 176MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.38G/8.31G [00:08<00:42, 175MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.40G/8.31G [00:08<00:42, 176MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.42G/8.31G [00:08<00:41, 179MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.44G/8.31G [00:08<00:40, 183MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.46G/8.31G [00:09<00:39, 186MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.47G/8.31G [00:09<00:38, 188MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.49G/8.31G [00:09<00:38, 189MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.51G/8.31G [00:09<00:38, 190MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.53G/8.31G [00:09<00:38, 190MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▊ | 1.55G/8.31G [00:09<00:38, 191MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▉ | 1.57G/8.31G [00:09<00:38, 189MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▉ | 1.59G/8.31G [00:09<00:38, 186MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▉ | 1.61G/8.31G [00:09<00:38, 185MB/s]
Downloading best_ckpt_mp0.tar: 20%|█▉ | 1.63G/8.31G [00:10<00:38, 188MB/s]
Downloading best_ckpt_mp0.tar: 20%|█▉ | 1.65G/8.31G [00:10<00:37, 189MB/s]
Downloading best_ckpt_mp0.tar: 20%|██ | 1.67G/8.31G [00:10<00:38, 187MB/s]
Downloading best_ckpt_mp0.tar: 20%|██ | 1.69G/8.31G [00:10<01:02, 114MB/s]
Downloading best_ckpt_mp0.tar: 21%|██ | 1.71G/8.31G [00:10<01:06, 107MB/s]
Downloading best_ckpt_mp0.tar: 21%|██ | 1.73G/8.31G [00:11<01:20, 87.4MB/s]
Downloading best_ckpt_mp0.tar: 21%|██ | 1.75G/8.31G [00:11<01:12, 96.8MB/s]
Downloading best_ckpt_mp0.tar: 21%|██▏ | 1.77G/8.31G [00:11<01:04, 109MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.79G/8.31G [00:11<01:08, 103MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.81G/8.31G [00:11<01:00, 115MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.83G/8.31G [00:12<00:54, 127MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.85G/8.31G [00:12<00:50, 137MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.87G/8.31G [00:12<00:54, 128MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.88G/8.31G [00:12<01:06, 103MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.90G/8.31G [00:12<01:22, 83.0MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.91G/8.31G [00:13<01:36, 71.2MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.92G/8.31G [00:13<01:37, 70.6MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.94G/8.31G [00:13<01:16, 89.1MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▎ | 1.96G/8.31G [00:13<01:06, 102MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▍ | 1.98G/8.31G [00:13<01:00, 113MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▍ | 2.00G/8.31G [00:13<00:53, 126MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▍ | 2.02G/8.31G [00:14<00:53, 126MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▍ | 2.04G/8.31G [00:14<00:51, 130MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▍ | 2.06G/8.31G [00:14<00:55, 120MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▌ | 2.08G/8.31G [00:14<01:04, 103MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▌ | 2.10G/8.31G [00:14<01:03, 105MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.12G/8.31G [00:15<01:02, 106MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.14G/8.31G [00:15<01:09, 96.0MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.15G/8.31G [00:15<01:14, 88.7MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.17G/8.31G [00:15<01:02, 106MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▋ | 2.19G/8.31G [00:15<00:55, 119MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.21G/8.31G [00:15<00:50, 130MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.23G/8.31G [00:16<00:47, 137MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.25G/8.31G [00:16<00:44, 146MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.27G/8.31G [00:16<00:41, 155MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.29G/8.31G [00:16<00:40, 160MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.30G/8.31G [00:16<00:39, 163MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.32G/8.31G [00:16<00:39, 163MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.34G/8.31G [00:16<00:38, 166MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.36G/8.31G [00:16<00:38, 166MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▊ | 2.38G/8.31G [00:17<00:38, 167MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.40G/8.31G [00:17<00:37, 168MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.42G/8.31G [00:17<00:37, 170MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.44G/8.31G [00:17<00:36, 171MB/s]
Downloading best_ckpt_mp0.tar: 30%|██▉ | 2.46G/8.31G [00:17<00:37, 169MB/s]
Downloading best_ckpt_mp0.tar: 30%|██▉ | 2.48G/8.31G [00:17<00:37, 167MB/s]
Downloading best_ckpt_mp0.tar: 30%|███ | 2.50G/8.31G [00:17<00:37, 167MB/s]
Downloading best_ckpt_mp0.tar: 30%|███ | 2.52G/8.31G [00:17<00:36, 169MB/s]
Downloading best_ckpt_mp0.tar: 31%|███ | 2.54G/8.31G [00:18<00:35, 173MB/s]
Downloading best_ckpt_mp0.tar: 31%|███ | 2.56G/8.31G [00:18<00:34, 177MB/s]
Downloading best_ckpt_mp0.tar: 31%|███ | 2.58G/8.31G [00:18<00:33, 182MB/s]
Downloading best_ckpt_mp0.tar: 31%|███▏ | 2.60G/8.31G [00:18<00:33, 181MB/s]
Downloading best_ckpt_mp0.tar: 31%|███▏ | 2.62G/8.31G [00:18<00:33, 183MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.64G/8.31G [00:18<00:32, 186MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.66G/8.31G [00:18<00:32, 187MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.68G/8.31G [00:18<00:32, 189MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.70G/8.31G [00:18<00:31, 190MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.71G/8.31G [00:19<00:31, 189MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.73G/8.31G [00:19<00:31, 189MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.75G/8.31G [00:19<00:32, 185MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.77G/8.31G [00:19<00:32, 184MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▎ | 2.79G/8.31G [00:19<00:32, 181MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▍ | 2.81G/8.31G [00:19<00:33, 178MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▍ | 2.83G/8.31G [00:19<00:32, 182MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▍ | 2.85G/8.31G [00:19<00:31, 185MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▍ | 2.87G/8.31G [00:19<00:31, 187MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▍ | 2.89G/8.31G [00:20<00:31, 187MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▌ | 2.91G/8.31G [00:20<00:31, 186MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▌ | 2.93G/8.31G [00:20<00:31, 184MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▌ | 2.95G/8.31G [00:20<00:31, 184MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▌ | 2.97G/8.31G [00:20<00:30, 185MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▌ | 2.99G/8.31G [00:20<00:30, 186MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▌ | 3.01G/8.31G [00:20<00:30, 186MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▋ | 3.03G/8.31G [00:20<00:30, 187MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.05G/8.31G [00:20<00:30, 186MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.07G/8.31G [00:21<00:30, 187MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.09G/8.31G [00:21<00:29, 191MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.11G/8.31G [00:21<00:29, 191MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.12G/8.31G [00:21<00:29, 191MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.14G/8.31G [00:21<00:28, 191MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.16G/8.31G [00:21<00:28, 191MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.18G/8.31G [00:21<00:29, 189MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▊ | 3.20G/8.31G [00:21<00:29, 189MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.22G/8.31G [00:21<00:29, 188MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.24G/8.31G [00:22<00:29, 184MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.26G/8.31G [00:22<00:30, 180MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.28G/8.31G [00:22<00:30, 176MB/s]
Downloading best_ckpt_mp0.tar: 40%|███▉ | 3.30G/8.31G [00:22<00:31, 173MB/s]
Downloading best_ckpt_mp0.tar: 40%|███▉ | 3.32G/8.31G [00:22<00:31, 170MB/s]
Downloading best_ckpt_mp0.tar: 40%|████ | 3.34G/8.31G [00:22<00:31, 169MB/s]
Downloading best_ckpt_mp0.tar: 40%|████ | 3.36G/8.31G [00:22<00:31, 168MB/s]
Downloading best_ckpt_mp0.tar: 41%|████ | 3.38G/8.31G [00:22<00:31, 168MB/s]
Downloading best_ckpt_mp0.tar: 41%|████ | 3.40G/8.31G [00:23<00:31, 170MB/s]
Downloading best_ckpt_mp0.tar: 41%|████ | 3.42G/8.31G [00:23<00:30, 172MB/s]
Downloading best_ckpt_mp0.tar: 41%|████▏ | 3.44G/8.31G [00:23<00:31, 168MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.46G/8.31G [00:23<00:31, 167MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.48G/8.31G [00:23<00:31, 167MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.50G/8.31G [00:23<00:30, 168MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.52G/8.31G [00:23<00:30, 169MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.54G/8.31G [00:23<00:30, 167MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.55G/8.31G [00:24<00:30, 169MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.57G/8.31G [00:24<00:29, 170MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.59G/8.31G [00:24<00:29, 171MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.61G/8.31G [00:24<00:28, 174MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▎ | 3.63G/8.31G [00:24<00:28, 177MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▍ | 3.65G/8.31G [00:24<00:28, 178MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▍ | 3.67G/8.31G [00:24<00:27, 180MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▍ | 3.69G/8.31G [00:24<00:27, 179MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▍ | 3.71G/8.31G [00:24<00:27, 180MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▍ | 3.73G/8.31G [00:25<00:27, 180MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▌ | 3.75G/8.31G [00:25<00:27, 179MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▌ | 3.77G/8.31G [00:25<00:27, 176MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▌ | 3.79G/8.31G [00:25<00:27, 177MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▌ | 3.81G/8.31G [00:25<00:27, 174MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▌ | 3.83G/8.31G [00:25<00:42, 112MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▋ | 3.85G/8.31G [00:26<00:52, 91.1MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.87G/8.31G [00:26<00:50, 94.2MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.89G/8.31G [00:26<00:48, 98.1MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.91G/8.31G [00:26<00:46, 101MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.93G/8.31G [00:27<01:01, 76.8MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.94G/8.31G [00:27<01:07, 69.7MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.96G/8.31G [00:27<00:56, 83.0MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.96G/8.31G [00:27<00:54, 86.1MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.97G/8.31G [00:28<01:07, 69.3MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.98G/8.31G [00:28<01:08, 68.2MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 4.00G/8.31G [00:28<00:53, 86.4MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 4.02G/8.31G [00:28<00:46, 98.6MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▊ | 4.04G/8.31G [00:28<00:54, 84.0MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.05G/8.31G [00:29<01:00, 75.4MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.07G/8.31G [00:29<00:49, 92.6MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.08G/8.31G [00:29<00:49, 91.1MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.10G/8.31G [00:29<00:49, 91.7MB/s]
Downloading best_ckpt_mp0.tar: 50%|████▉ | 4.12G/8.31G [00:29<00:41, 109MB/s]
Downloading best_ckpt_mp0.tar: 50%|████▉ | 4.14G/8.31G [00:29<00:42, 105MB/s]
Downloading best_ckpt_mp0.tar: 50%|█████ | 4.16G/8.31G [00:30<00:54, 81.2MB/s]
Downloading best_ckpt_mp0.tar: 50%|█████ | 4.17G/8.31G [00:30<00:56, 78.2MB/s]
Downloading best_ckpt_mp0.tar: 50%|█████ | 4.19G/8.31G [00:30<00:47, 92.8MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████ | 4.21G/8.31G [00:30<00:39, 112MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████ | 4.23G/8.31G [00:30<00:34, 128MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████ | 4.25G/8.31G [00:30<00:31, 140MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████▏ | 4.27G/8.31G [00:30<00:28, 152MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.29G/8.31G [00:31<00:26, 161MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.31G/8.31G [00:31<00:26, 164MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.33G/8.31G [00:31<00:25, 166MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.35G/8.31G [00:31<00:24, 172MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.37G/8.31G [00:31<00:24, 176MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.38G/8.31G [00:31<00:23, 180MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.40G/8.31G [00:31<00:22, 184MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.42G/8.31G [00:31<00:22, 188MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.44G/8.31G [00:31<00:21, 189MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▎ | 4.46G/8.31G [00:32<00:22, 187MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▍ | 4.48G/8.31G [00:32<00:21, 187MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▍ | 4.50G/8.31G [00:32<00:21, 186MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▍ | 4.52G/8.31G [00:32<00:21, 186MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▍ | 4.54G/8.31G [00:32<00:21, 185MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▍ | 4.56G/8.31G [00:32<00:21, 186MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▌ | 4.58G/8.31G [00:32<00:21, 186MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▌ | 4.60G/8.31G [00:32<00:24, 163MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▌ | 4.62G/8.31G [00:33<00:24, 164MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▌ | 4.64G/8.31G [00:33<00:23, 166MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▌ | 4.66G/8.31G [00:33<00:22, 174MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▋ | 4.68G/8.31G [00:33<00:22, 177MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.70G/8.31G [00:33<00:22, 176MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.72G/8.31G [00:33<00:22, 171MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.74G/8.31G [00:33<00:22, 169MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.76G/8.31G [00:33<00:22, 170MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.78G/8.31G [00:34<00:22, 170MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.79G/8.31G [00:34<00:22, 167MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.81G/8.31G [00:34<00:22, 166MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.83G/8.31G [00:34<00:22, 165MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.85G/8.31G [00:34<00:22, 165MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▊ | 4.87G/8.31G [00:34<00:22, 165MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▉ | 4.89G/8.31G [00:34<00:22, 162MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▉ | 4.91G/8.31G [00:34<00:22, 162MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▉ | 4.93G/8.31G [00:35<00:22, 163MB/s]
Downloading best_ckpt_mp0.tar: 60%|█████▉ | 4.95G/8.31G [00:35<00:21, 167MB/s]
Downloading best_ckpt_mp0.tar: 60%|█████▉ | 4.97G/8.31G [00:35<00:21, 170MB/s]
Downloading best_ckpt_mp0.tar: 60%|██████ | 4.99G/8.31G [00:35<00:20, 172MB/s]
Downloading best_ckpt_mp0.tar: 60%|██████ | 5.01G/8.31G [00:35<00:20, 170MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.03G/8.31G [00:35<00:20, 172MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.05G/8.31G [00:35<00:20, 169MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.07G/8.31G [00:35<00:20, 170MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.09G/8.31G [00:36<00:20, 169MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████▏ | 5.11G/8.31G [00:36<00:20, 169MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.13G/8.31G [00:36<00:19, 172MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.15G/8.31G [00:36<00:19, 176MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.17G/8.31G [00:36<00:18, 178MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.19G/8.31G [00:36<00:18, 179MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.21G/8.31G [00:36<00:18, 180MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.22G/8.31G [00:36<00:18, 180MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.24G/8.31G [00:36<00:18, 177MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.26G/8.31G [00:37<00:18, 176MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▎ | 5.28G/8.31G [00:37<00:18, 179MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▍ | 5.30G/8.31G [00:37<00:17, 181MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▍ | 5.32G/8.31G [00:37<00:17, 180MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▍ | 5.34G/8.31G [00:37<00:17, 178MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▍ | 5.36G/8.31G [00:37<00:17, 179MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▍ | 5.38G/8.31G [00:37<00:17, 179MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▍ | 5.40G/8.31G [00:37<00:17, 179MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▌ | 5.42G/8.31G [00:38<00:17, 176MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▌ | 5.44G/8.31G [00:38<00:17, 173MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▌ | 5.46G/8.31G [00:38<00:17, 171MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▌ | 5.48G/8.31G [00:38<00:17, 172MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▌ | 5.50G/8.31G [00:38<00:17, 170MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▋ | 5.52G/8.31G [00:38<00:17, 168MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.54G/8.31G [00:38<00:17, 169MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.56G/8.31G [00:38<00:17, 171MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.58G/8.31G [00:39<00:16, 176MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.60G/8.31G [00:39<00:16, 179MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.62G/8.31G [00:39<00:16, 181MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.63G/8.31G [00:39<00:15, 182MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.65G/8.31G [00:39<00:15, 180MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.67G/8.31G [00:39<00:15, 179MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▊ | 5.69G/8.31G [00:39<00:16, 174MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.71G/8.31G [00:39<00:16, 171MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.73G/8.31G [00:39<00:16, 170MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.75G/8.31G [00:40<00:16, 168MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.77G/8.31G [00:40<00:16, 168MB/s]
Downloading best_ckpt_mp0.tar: 70%|██████▉ | 5.79G/8.31G [00:40<00:16, 168MB/s]
Downloading best_ckpt_mp0.tar: 70%|██████▉ | 5.81G/8.31G [00:40<00:15, 169MB/s]
Downloading best_ckpt_mp0.tar: 70%|███████ | 5.83G/8.31G [00:40<00:17, 155MB/s]
Downloading best_ckpt_mp0.tar: 70%|███████ | 5.85G/8.31G [00:40<00:21, 122MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████ | 5.87G/8.31G [00:41<00:28, 92.9MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████ | 5.89G/8.31G [00:41<00:24, 106MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████ | 5.91G/8.31G [00:41<00:23, 109MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████▏ | 5.93G/8.31G [00:41<00:25, 101MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.95G/8.31G [00:42<00:35, 72.3MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.96G/8.31G [00:42<00:36, 69.2MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.97G/8.31G [00:42<00:37, 66.4MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.99G/8.31G [00:42<00:29, 84.6MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 6.01G/8.31G [00:43<00:27, 88.6MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 6.02G/8.31G [00:43<00:34, 71.5MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.03G/8.31G [00:43<00:35, 69.9MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.04G/8.31G [00:43<00:27, 89.4MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.06G/8.31G [00:43<00:22, 106MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.08G/8.31G [00:43<00:20, 115MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.10G/8.31G [00:43<00:18, 126MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▎ | 6.12G/8.31G [00:44<00:22, 102MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▍ | 6.14G/8.31G [00:44<00:20, 113MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▍ | 6.16G/8.31G [00:44<00:20, 112MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▍ | 6.18G/8.31G [00:44<00:27, 84.2MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▍ | 6.19G/8.31G [00:45<00:28, 81.1MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▍ | 6.20G/8.31G [00:45<00:26, 84.0MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▍ | 6.22G/8.31G [00:45<00:21, 103MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▌ | 6.24G/8.31G [00:45<00:20, 109MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▌ | 6.26G/8.31G [00:45<00:17, 123MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▌ | 6.28G/8.31G [00:45<00:15, 136MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▌ | 6.30G/8.31G [00:45<00:14, 147MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▌ | 6.32G/8.31G [00:46<00:13, 155MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▋ | 6.34G/8.31G [00:46<00:13, 163MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.36G/8.31G [00:46<00:12, 168MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.38G/8.31G [00:46<00:12, 173MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.40G/8.31G [00:46<00:11, 176MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.42G/8.31G [00:46<00:11, 177MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.44G/8.31G [00:46<00:11, 178MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.46G/8.31G [00:46<00:11, 176MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.47G/8.31G [00:46<00:11, 172MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.49G/8.31G [00:47<00:11, 169MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.51G/8.31G [00:47<00:11, 170MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▊ | 6.53G/8.31G [00:47<00:11, 171MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▉ | 6.55G/8.31G [00:47<00:10, 172MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▉ | 6.57G/8.31G [00:47<00:10, 176MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▉ | 6.59G/8.31G [00:47<00:10, 177MB/s]
Downloading best_ckpt_mp0.tar: 80%|███████▉ | 6.61G/8.31G [00:47<00:10, 178MB/s]
Downloading best_ckpt_mp0.tar: 80%|███████▉ | 6.63G/8.31G [00:47<00:10, 178MB/s]
Downloading best_ckpt_mp0.tar: 80%|████████ | 6.65G/8.31G [00:48<00:10, 177MB/s]
Downloading best_ckpt_mp0.tar: 80%|████████ | 6.67G/8.31G [00:48<00:09, 177MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.69G/8.31G [00:48<00:09, 178MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.71G/8.31G [00:48<00:09, 179MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.73G/8.31G [00:48<00:09, 180MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.75G/8.31G [00:48<00:09, 180MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████▏ | 6.77G/8.31G [00:48<00:09, 179MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.79G/8.31G [00:48<00:09, 177MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.81G/8.31G [00:48<00:09, 175MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.83G/8.31G [00:49<00:09, 170MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.85G/8.31G [00:49<00:09, 170MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.87G/8.31G [00:49<00:09, 172MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.88G/8.31G [00:49<00:08, 176MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.90G/8.31G [00:49<00:08, 178MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.92G/8.31G [00:49<00:08, 180MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▎ | 6.94G/8.31G [00:49<00:08, 179MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▍ | 6.96G/8.31G [00:49<00:08, 177MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▍ | 6.98G/8.31G [00:50<00:08, 175MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▍ | 7.00G/8.31G [00:50<00:08, 175MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▍ | 7.02G/8.31G [00:50<00:08, 173MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▍ | 7.04G/8.31G [00:50<00:07, 173MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▍ | 7.06G/8.31G [00:50<00:07, 172MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▌ | 7.08G/8.31G [00:50<00:07, 170MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▌ | 7.10G/8.31G [00:50<00:07, 170MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▌ | 7.12G/8.31G [00:50<00:07, 168MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▌ | 7.14G/8.31G [00:51<00:07, 166MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▌ | 7.16G/8.31G [00:51<00:07, 170MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▋ | 7.18G/8.31G [00:51<00:07, 170MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.20G/8.31G [00:51<00:06, 172MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.22G/8.31G [00:51<00:06, 173MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.24G/8.31G [00:51<00:06, 174MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.26G/8.31G [00:51<00:06, 176MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.28G/8.31G [00:51<00:06, 177MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.29G/8.31G [00:52<00:06, 180MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.31G/8.31G [00:52<00:05, 183MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.33G/8.31G [00:52<00:05, 184MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.35G/8.31G [00:52<00:05, 184MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▊ | 7.37G/8.31G [00:52<00:05, 184MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▉ | 7.39G/8.31G [00:52<00:05, 182MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▉ | 7.41G/8.31G [00:52<00:05, 182MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▉ | 7.43G/8.31G [00:52<00:05, 182MB/s]
Downloading best_ckpt_mp0.tar: 90%|████████▉ | 7.45G/8.31G [00:52<00:05, 183MB/s]
Downloading best_ckpt_mp0.tar: 90%|████████▉ | 7.47G/8.31G [00:53<00:04, 181MB/s]
Downloading best_ckpt_mp0.tar: 90%|█████████ | 7.49G/8.31G [00:53<00:04, 180MB/s]
Downloading best_ckpt_mp0.tar: 90%|█████████ | 7.51G/8.31G [00:53<00:04, 181MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████ | 7.53G/8.31G [00:53<00:04, 181MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████ | 7.55G/8.31G [00:53<00:04, 182MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████ | 7.57G/8.31G [00:53<00:04, 182MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████▏| 7.59G/8.31G [00:53<00:04, 181MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.61G/8.31G [00:53<00:04, 180MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.63G/8.31G [00:53<00:04, 179MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.65G/8.31G [00:54<00:04, 175MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.67G/8.31G [00:54<00:03, 175MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.69G/8.31G [00:54<00:03, 175MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.71G/8.31G [00:54<00:03, 178MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.72G/8.31G [00:54<00:03, 180MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.74G/8.31G [00:54<00:03, 182MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.76G/8.31G [00:54<00:03, 184MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▎| 7.78G/8.31G [00:54<00:03, 185MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▍| 7.80G/8.31G [00:55<00:02, 186MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▍| 7.82G/8.31G [00:55<00:02, 186MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▍| 7.84G/8.31G [00:55<00:02, 184MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▍| 7.86G/8.31G [00:55<00:02, 184MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▍| 7.88G/8.31G [00:55<00:02, 184MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▌| 7.90G/8.31G [00:55<00:03, 131MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▌| 7.92G/8.31G [00:56<00:05, 81.1MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.94G/8.31G [00:56<00:05, 76.6MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.96G/8.31G [00:56<00:04, 90.7MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.98G/8.31G [00:57<00:04, 75.1MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.99G/8.31G [00:57<00:04, 71.3MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▋| 8.00G/8.31G [00:57<00:05, 61.6MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▋| 8.01G/8.31G [00:57<00:05, 59.9MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.03G/8.31G [00:57<00:04, 69.9MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.04G/8.31G [00:58<00:04, 62.9MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.05G/8.31G [00:58<00:04, 56.7MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.07G/8.31G [00:58<00:03, 77.1MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.08G/8.31G [00:58<00:03, 78.5MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.10G/8.31G [00:58<00:02, 77.1MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.11G/8.31G [00:59<00:02, 79.3MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.12G/8.31G [00:59<00:02, 92.2MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.14G/8.31G [00:59<00:02, 73.9MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.15G/8.31G [00:59<00:02, 68.7MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.16G/8.31G [00:59<00:02, 67.6MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.18G/8.31G [01:00<00:01, 88.4MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▊| 8.20G/8.31G [01:00<00:01, 106MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▉| 8.22G/8.31G [01:00<00:00, 123MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▉| 8.24G/8.31G [01:00<00:00, 137MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▉| 8.26G/8.31G [01:00<00:00, 150MB/s]
Downloading best_ckpt_mp0.tar: 100%|█████████▉| 8.28G/8.31G [01:00<00:00, 161MB/s]
Downloading best_ckpt_mp0.tar: 100%|█████████▉| 8.30G/8.31G [01:00<00:00, 169MB/s]
Downloading best_ckpt_mp0.tar: 100%|██████████| 8.31G/8.31G [01:00<00:00, 147MB/s]
2025-05-16 00:33:04.666 | INFO | earth2studio.run:ensemble:315 - Running ensemble inference!
2025-05-16 00:33:04.666 | INFO | earth2studio.run:ensemble:323 - Inference device: cuda
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.925 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 354558976-950206
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.946 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268038708-839499
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.946 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 165935718-1138677
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.947 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204839116-753955
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.947 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 169090127-920171
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.948 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 350072351-1232650
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.949 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 353619033-939943
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.949 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 180172685-913532
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.950 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 387322476-948106
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.974 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 289307267-851916
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.997 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 176434139-1041731
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.998 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 226457235-723507
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:05.999 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 406629528-962408
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.022 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 383286081-1212507
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.023 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 274285343-895314
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.023 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 329739828-930772
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.047 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 190027998-577814
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.048 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 143527711-755695
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.071 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323061199-895080
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.094 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 186591756-1053680
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.095 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 247139652-717479
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.118 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 149106074-462399
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.119 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 414179964-1179422
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.142 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199934883-595444
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.164 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 163781343-739174
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.165 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 346879212-932889
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.166 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 196462617-1008822
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.166 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268878207-724790
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.167 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 0-993995
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.189 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 386359113-963363
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.212 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 404382959-913875
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.213 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210820017-615282
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.213 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174160271-736006
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.214 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 391722290-987401
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.237 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 206944850-1065961
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.237 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 290159183-752827
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.238 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 295659093-896559
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.239 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 452597070-961302
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.261 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 232400432-586007
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.262 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184050412-731750
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.263 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 144283406-743766
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.263 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 228474082-1138597
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.264 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323956279-837771
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.287 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 148613430-492644
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.288 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 402321768-876246
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.311 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 253514796-920355
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.334 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 193737151-726758
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.357 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 164520517-765194
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.358 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 249098202-1262404
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.358 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 347812101-849637
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.359 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 231827443-572989
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.360 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 451628742-968328
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.382 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 275180657-890286
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.383 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204118947-720169
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.405 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174896277-746582
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.406 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 270785488-1177846
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.406 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 381424372-852801
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.407 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210217024-602993
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.408 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 252556659-958137
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.430 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 296555652-890544
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.431 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 225640684-816551
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.431 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184782162-755841
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.432 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 292088625-1244938
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.433 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 145318799-1019625
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.433 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 393705863-838502
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.456 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 179198897-973788
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.456 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 407591936-940269
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.479 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 330670600-938837
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.502 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 246334297-805355
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.525 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 194463909-743465
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.547 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 326166249-1225442
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.548 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199346060-588823
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.571 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 189450163-577835
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:33:06.572 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 170010298-904882
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
Fetching GFS data: 1%|▏ | 1/74 [00:00<00:47, 1.54it/s]
Fetching GFS data: 36%|███▋ | 27/74 [00:01<00:02, 22.88it/s]
Fetching GFS data: 41%|████ | 30/74 [00:01<00:01, 22.55it/s]
Fetching GFS data: 50%|█████ | 37/74 [00:01<00:01, 28.61it/s]
Fetching GFS data: 57%|█████▋ | 42/74 [00:01<00:01, 28.73it/s]
Fetching GFS data: 64%|██████▎ | 47/74 [00:01<00:00, 32.27it/s]
Fetching GFS data: 70%|███████ | 52/74 [00:01<00:00, 35.14it/s]
Fetching GFS data: 77%|███████▋ | 57/74 [00:02<00:00, 29.78it/s]
Fetching GFS data: 86%|████████▋ | 64/74 [00:02<00:00, 33.91it/s]
Fetching GFS data: 96%|█████████▌| 71/74 [00:02<00:00, 39.19it/s]
Fetching GFS data: 100%|██████████| 74/74 [00:02<00:00, 28.97it/s]
2025-05-16 00:33:08.684 | SUCCESS | earth2studio.run:ensemble:345 - Fetched data from GFS
2025-05-16 00:33:08.690 | WARNING | earth2studio.io.zarr:add_array:192 - Datetime64 not supported in zarr 3.0, converting to int64 nanoseconds since epoch
2025-05-16 00:33:08.693 | WARNING | earth2studio.io.zarr:add_array:198 - Timedelta64 not supported in zarr 3.0, converting to int64 nanoseconds since epoch
2025-05-16 00:33:08.706 | INFO | earth2studio.run:ensemble:373 - Starting 2 Member Ensemble Inference with 2 number of batches.
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
2025-05-16 00:33:09.141 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 411298483-922437
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.143 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 199460585-743054
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.145 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 273976632-728286
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.147 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 381424372-852801
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.188 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 333255379-930566
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.189 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 215757651-606283
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.190 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 301084617-899856
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.191 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 149106074-462399
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.214 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 459841403-963517
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.216 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 210135611-756844
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.217 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 295547922-758800
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.217 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 145318799-1019625
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.241 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 334185945-934655
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.243 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 237907755-575171
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.243 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 334970306-938022
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.244 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 170010298-904882
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.268 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 152040961-493376
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.270 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 232267599-726560
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.270 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 329116923-847018
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.294 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 165935718-1138677
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.318 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 257413354-553231
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.320 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 259087914-916036
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.320 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 359046224-949853
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.321 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 180172685-913532
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.345 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 387152087-1211521
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.346 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 253521451-719382
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.347 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 279542923-898410
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.348 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 353190489-858785
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.348 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 176434139-1041731
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.373 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 183507493-913609
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.374 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 281281791-898336
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.375 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 392065452-969982
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.375 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 190027998-577814
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.399 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 421300034-1181973
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.399 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 275795645-726593
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.400 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 387075654-860051
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.401 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 186591756-1053680
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.425 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 193820670-578278
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.426 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 302819301-896743
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.427 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 150898120-463977
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.428 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199934883-595444
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.455 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 253388728-1269329
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.456 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 297291574-754222
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.457 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 147098312-1020507
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.457 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 196462617-1008822
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.481 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 177186467-735476
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.482 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 336687879-934577
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.482 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 172227940-905153
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.483 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210820017-615282
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.506 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 330695860-846471
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.507 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 167747263-1134797
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.508 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 206944850-1065961
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.530 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 214779600-623304
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.531 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 360780804-942376
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.532 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 183377778-912918
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.533 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 232400432-586007
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.556 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 168789447-980831
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.557 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 354932182-856465
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.558 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 179108822-1168912
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.558 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 228474082-1138597
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.582 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 204088265-596882
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.583 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 393767669-968511
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.584 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 193675823-578990
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.585 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 253514796-920355
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.608 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 203498423-589842
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.609 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 327423370-840295
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.610 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 388773222-859658
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.611 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 189840662-1179194
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.611 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 249098202-1262404
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.636 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 357316538-939003
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.637 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 152534337-466715
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.638 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 203992655-596596
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.639 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 275180657-890286
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.662 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 295820475-1242797
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.662 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 148724824-1026834
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.663 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 200082859-1168749
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.664 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 270785488-1177846
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.687 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 151904457-465264
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.688 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 173902504-907905
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.689 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 215007021-618021
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.690 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 296555652-890544
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:33:09.713 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 271656637-839000
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.714 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 169411507-1139754
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.715 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 210882735-1186600
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.715 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 292088625-1244938
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.739 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 236647942-590553
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.740 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 184623371-911795
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.741 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 237104567-587286
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.742 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 330670600-938837
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.765 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 401150630-841557
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.766 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 180472580-1170586
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.767 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 232918171-1262978
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.768 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 326166249-1225442
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.793 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 456116956-963873
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.794 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 194932669-578572
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.795 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 258516328-918758
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.795 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 354558976-950206
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.818 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 179476202-1048997
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.819 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 191094271-1176950
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.820 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 254001564-1267820
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.821 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 350072351-1232650
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.844 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 455143487-973469
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.845 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 205351099-596883
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.846 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 280441333-889661
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.847 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 387322476-948106
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.870 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 407776106-914088
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.871 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 208928508-761990
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.871 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 201444244-1166535
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.872 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 275899947-1309015
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.873 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 383286081-1212507
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.896 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 397639332-840040
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.897 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 216363934-618291
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.898 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 301984473-889315
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.899 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 143527711-755695
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.922 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 198184389-747202
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.923 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 212236463-1187155
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.923 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 297490896-1247432
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.924 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 0-1031836
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.925 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 238482926-588964
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.926 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 335908328-940530
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.926 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 163781343-739174
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.949 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 293912488-745515
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.950 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 234294213-1264469
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.951 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 331362340-1232012
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.952 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 417602460-1179045
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.952 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 260003950-922575
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.953 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 359996077-949678
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.954 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174160271-736006
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.977 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 204760966-590133
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.978 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 255488233-1268872
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.978 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 355472229-1239204
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.979 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 172072425-918801
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.980 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 282180127-893274
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.981 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 393035434-953486
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:09.981 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184050412-731750
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.005 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 326532594-890776
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.006 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 274408443-1223885
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.007 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 277722016-1226189
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.007 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 388958699-1221367
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.008 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 151411717-492740
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.009 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 303716044-887380
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.009 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 145304873-758046
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.010 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 193737151-726758
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.034 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 293067730-844758
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.035 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 200175442-1167472
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.035 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 299224343-1245614
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.036 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 406629528-962408
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.059 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 410990905-942963
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.060 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 337622456-936189
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.061 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 165594251-738958
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.062 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204118947-720169
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.085 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 250614479-803472
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.086 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 172983015-919489
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.087 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 332945430-1359233
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.088 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 407591936-940269
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.111 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 390243670-963082
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.112 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 361723180-950913
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.113 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 176832054-736857
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.113 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 225640684-816551
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.137 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 148091792-1031496
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.138 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 357218775-1228059
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.139 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 451628742-968328
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.162 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 172991226-911247
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.163 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 394736180-951384
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.164 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 187292486-732506
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.164 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 246334297-805355
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.188 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 395625686-986980
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.189 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 390660597-1219295
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.190 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 452597070-961302
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.213 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 214171943-607657
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.214 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 146927534-759351
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.214 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 197360802-728078
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.215 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268038708-839499
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.239 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 300299872-884864
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.240 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 183650695-972676
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.241 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 412411490-969112
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.242 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 402321768-876246
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.265 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 277968948-896544
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.266 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 167248172-739678
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.267 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 208052937-721817
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.290 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 289307267-851916
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.313 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 278865492-888548
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.314 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 351476990-852387
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.315 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 413380602-945786
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.316 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 393705863-838502
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.339 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 299407315-892557
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.340 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 178183898-738189
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.341 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 230071239-818111
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.342 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323061199-895080
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.365 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 166625127-737840
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.366 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 329654990-1225009
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.367 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 457464510-979481
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.368 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 0-993995
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.391 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 193241168-579502
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.392 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 188547657-732362
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.393 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 251230645-803982
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.421 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 346879212-932889
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.445 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 182534428-973065
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.446 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 353761167-1225423
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.447 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 458443991-962968
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.448 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 414179964-1179422
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.472 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 198733171-727414
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.473 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 273134252-842380
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.474 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 391722290-987401
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.498 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 350547115-929875
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.499 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 194353089-579580
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.500 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 408062467-879185
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.525 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 404382959-913875
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.549 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 209414924-720687
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.550 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 294691465-856457
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.574 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 144283406-743766
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.597 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 197458039-726350
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.598 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 211043451-1192232
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.598 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 399434135-840126
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.599 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 148613430-492644
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.622 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 231450583-817016
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.623 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 328218119-898804
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.624 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 386359113-963363
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.649 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 164520517-765194
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.674 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 208203900-724608
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.675 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 0-1002356
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.676 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 169090127-920171
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.700 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 252718154-803297
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.701 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 352251507-938982
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.702 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174896277-746582
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:33:10.725 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 414536832-944207
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.726 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 229726800-817683
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.727 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 420029701-1181204
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.751 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 179198897-973788
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.774 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 410028724-962181
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.776 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 274954839-840806
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.776 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 397402829-996456
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.802 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184782162-755841
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.826 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 187427126-731084
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.827 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 391206752-950631
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.828 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 409589953-513282
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.829 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 410138186-925503
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.830 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 189450163-577835
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.855 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 236071718-576224
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.856 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 296442783-848791
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.856 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 146062919-744726
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.857 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 194463909-743465
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.880 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 146298066-757068
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.881 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 272495637-727089
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.882 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 150405231-492889
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.883 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199346060-588823
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.905 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 329799511-896349
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.907 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 166333209-766399
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.907 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204839116-753955
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.930 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 188158210-757568
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.932 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 232576858-1266241
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.932 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 171310346-917594
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.933 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210217024-602993
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.956 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 256868469-544885
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.957 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 353999639-932543
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.958 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 177568911-747761
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.959 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 226457235-723507
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.983 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 358255541-950771
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.984 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 413569354-967478
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.985 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 182403895-973883
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:10.986 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 231827443-572989
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.010 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 399119260-992978
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.012 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 188024992-754829
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.012 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 247139652-717479
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.036 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 406073657-506593
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.037 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 385279214-852759
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.038 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 193097116-578707
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.039 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 252556659-958137
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.062 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 147686885-749156
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.063 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 198088880-741667
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.064 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268878207-724790
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.087 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 167362967-767433
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.088 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 189979250-1176980
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.089 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 203402796-589859
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.090 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 274285343-895314
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.113 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 167987850-767526
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.114 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 208774754-755459
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.115 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 290159183-752827
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.138 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 177921943-752161
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.139 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 458864763-976640
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.140 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 214401793-605228
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.141 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 295659093-896559
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.165 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 178922087-749074
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.166 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 230889350-725457
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.167 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323956279-837771
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.191 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 251417951-723202
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.192 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 236529889-574678
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.193 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 329739828-930772
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.217 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 189280019-753310
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.218 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 252034627-722839
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.219 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 347812101-849637
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.243 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 147055134-746039
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.244 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 0-998436
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.245 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 257599990-916338
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.246 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 353619033-939943
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:33:11.270 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 230544483-732296
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
Fetching GFS data: 0%| | 1/296 [00:02<10:29, 2.13s/it]
Fetching GFS data: 28%|██▊ | 82/296 [00:02<00:04, 48.44it/s]
Fetching GFS data: 36%|███▌ | 107/296 [00:03<00:04, 37.91it/s]
Fetching GFS data: 41%|████ | 122/296 [00:03<00:04, 37.30it/s]
Fetching GFS data: 45%|████▍ | 133/296 [00:04<00:04, 37.88it/s]
Fetching GFS data: 48%|████▊ | 142/296 [00:04<00:04, 37.54it/s]
Fetching GFS data: 50%|█████ | 149/296 [00:04<00:03, 38.54it/s]
Fetching GFS data: 52%|█████▏ | 155/296 [00:04<00:03, 38.80it/s]
Fetching GFS data: 54%|█████▍ | 161/296 [00:04<00:03, 40.12it/s]
Fetching GFS data: 56%|█████▋ | 167/296 [00:04<00:03, 41.50it/s]
Fetching GFS data: 58%|█████▊ | 172/296 [00:04<00:03, 39.25it/s]
Fetching GFS data: 60%|█████▉ | 177/296 [00:05<00:03, 38.12it/s]
Fetching GFS data: 61%|██████▏ | 182/296 [00:05<00:02, 38.42it/s]
Fetching GFS data: 63%|██████▎ | 187/296 [00:05<00:02, 40.55it/s]
Fetching GFS data: 65%|██████▍ | 192/296 [00:05<00:02, 37.86it/s]
Fetching GFS data: 66%|██████▌ | 196/296 [00:05<00:02, 38.21it/s]
Fetching GFS data: 68%|██████▊ | 200/296 [00:05<00:02, 38.56it/s]
Fetching GFS data: 69%|██████▉ | 205/296 [00:05<00:02, 36.50it/s]
Fetching GFS data: 71%|███████▏ | 211/296 [00:05<00:02, 42.22it/s]
Fetching GFS data: 73%|███████▎ | 216/296 [00:06<00:01, 41.63it/s]
Fetching GFS data: 75%|███████▍ | 221/296 [00:06<00:01, 38.75it/s]
Fetching GFS data: 76%|███████▋ | 226/296 [00:06<00:01, 39.21it/s]
Fetching GFS data: 78%|███████▊ | 231/296 [00:06<00:01, 41.84it/s]
Fetching GFS data: 80%|███████▉ | 236/296 [00:06<00:01, 41.18it/s]
Fetching GFS data: 81%|████████▏ | 241/296 [00:06<00:01, 36.13it/s]
Fetching GFS data: 83%|████████▎ | 247/296 [00:06<00:01, 35.70it/s]
Fetching GFS data: 86%|████████▌ | 254/296 [00:07<00:01, 34.06it/s]
Fetching GFS data: 89%|████████▉ | 264/296 [00:07<00:00, 36.62it/s]
Fetching GFS data: 93%|█████████▎| 274/296 [00:07<00:00, 36.91it/s]
Fetching GFS data: 96%|█████████▌| 283/296 [00:07<00:00, 37.19it/s]
Fetching GFS data: 99%|█████████▉| 293/296 [00:08<00:00, 45.69it/s]
Fetching GFS data: 100%|██████████| 296/296 [00:08<00:00, 36.85it/s]
Running batch 0 inference: 0%| | 0/5 [00:00<?, ?it/s]
Running batch 0 inference: 20%|██ | 1/5 [00:00<00:00, 4.54it/s]
Running batch 0 inference: 40%|████ | 2/5 [00:01<00:02, 1.15it/s]
Running batch 0 inference: 60%|██████ | 3/5 [00:02<00:02, 1.06s/it]
Running batch 0 inference: 80%|████████ | 4/5 [00:04<00:01, 1.17s/it]
Running batch 0 inference: 100%|██████████| 5/5 [00:05<00:00, 1.22s/it]
Total Ensemble Batches: 50%|█████ | 1/2 [00:27<00:27, 27.34s/it]
Running batch 1 inference: 0%| | 0/5 [00:00<?, ?it/s]
Running batch 1 inference: 20%|██ | 1/5 [00:00<00:00, 4.57it/s]
Running batch 1 inference: 40%|████ | 2/5 [00:01<00:02, 1.16it/s]
Running batch 1 inference: 60%|██████ | 3/5 [00:02<00:02, 1.07s/it]
Running batch 1 inference: 80%|████████ | 4/5 [00:04<00:01, 1.16s/it]
Running batch 1 inference: 100%|██████████| 5/5 [00:05<00:00, 1.21s/it]
Total Ensemble Batches: 100%|██████████| 2/2 [00:32<00:00, 14.46s/it]
Total Ensemble Batches: 100%|██████████| 2/2 [00:32<00:00, 16.39s/it]
2025-05-16 00:33:41.484 | SUCCESS | earth2studio.run:ensemble:423 - Inference complete
/
├── ensemble (2,) int64
├── lat (721,) float64
├── lead_time (5,) int64
├── lon (1440,) float64
├── time (1,) int64
├── u10m (2, 1, 5, 721, 1440) float32
└── v10m (2, 1, 5, 721, 1440) float32
Downloading config.json: 0%| | 0.00/22.4k [00:00<?, ?B/s]
Downloading config.json: 100%|██████████| 22.4k/22.4k [00:00<00:00, 221kB/s]
Downloading config.json: 100%|██████████| 22.4k/22.4k [00:00<00:00, 219kB/s]
Downloading orography.nc: 0%| | 0.00/2.50M [00:00<?, ?B/s]
Downloading orography.nc: 100%|██████████| 2.50M/2.50M [00:00<00:00, 19.4MB/s]
Downloading orography.nc: 100%|██████████| 2.50M/2.50M [00:00<00:00, 19.1MB/s]
Downloading land_mask.nc: 0%| | 0.00/748k [00:00<?, ?B/s]
Downloading land_mask.nc: 100%|██████████| 748k/748k [00:00<00:00, 5.89MB/s]
Downloading land_mask.nc: 100%|██████████| 748k/748k [00:00<00:00, 5.78MB/s]
Downloading global_means.npy: 0%| | 0.00/720 [00:00<?, ?B/s]
Downloading global_means.npy: 100%|██████████| 720/720 [00:00<00:00, 5.99kB/s]
Downloading global_means.npy: 100%|██████████| 720/720 [00:00<00:00, 5.93kB/s]
Downloading global_stds.npy: 0%| | 0.00/720 [00:00<?, ?B/s]
Downloading global_stds.npy: 100%|██████████| 720/720 [00:00<00:00, 5.64kB/s]
Downloading global_stds.npy: 100%|██████████| 720/720 [00:00<00:00, 5.58kB/s]
Downloading best_ckpt_mp0.tar: 0%| | 0.00/8.31G [00:00<?, ?B/s]
Downloading best_ckpt_mp0.tar: 0%| | 10.0M/8.31G [00:00<05:52, 25.3MB/s]
Downloading best_ckpt_mp0.tar: 0%| | 30.0M/8.31G [00:00<02:11, 67.5MB/s]
Downloading best_ckpt_mp0.tar: 1%| | 50.0M/8.31G [00:00<01:31, 96.4MB/s]
Downloading best_ckpt_mp0.tar: 1%| | 70.0M/8.31G [00:00<01:14, 118MB/s]
Downloading best_ckpt_mp0.tar: 1%| | 90.0M/8.31G [00:00<01:06, 133MB/s]
Downloading best_ckpt_mp0.tar: 1%|▏ | 110M/8.31G [00:01<01:00, 146MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 130M/8.31G [00:01<00:56, 156MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 150M/8.31G [00:01<00:53, 164MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 170M/8.31G [00:01<00:51, 170MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 190M/8.31G [00:01<00:50, 174MB/s]
Downloading best_ckpt_mp0.tar: 2%|▏ | 210M/8.31G [00:01<00:49, 177MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 230M/8.31G [00:01<00:48, 179MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 250M/8.31G [00:01<00:48, 179MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 270M/8.31G [00:01<00:48, 179MB/s]
Downloading best_ckpt_mp0.tar: 3%|▎ | 290M/8.31G [00:02<00:48, 180MB/s]
Downloading best_ckpt_mp0.tar: 4%|▎ | 310M/8.31G [00:02<00:47, 181MB/s]
Downloading best_ckpt_mp0.tar: 4%|▍ | 330M/8.31G [00:02<00:47, 182MB/s]
Downloading best_ckpt_mp0.tar: 4%|▍ | 350M/8.31G [00:02<00:47, 181MB/s]
Downloading best_ckpt_mp0.tar: 4%|▍ | 370M/8.31G [00:02<00:47, 179MB/s]
Downloading best_ckpt_mp0.tar: 5%|▍ | 390M/8.31G [00:02<00:47, 179MB/s]
Downloading best_ckpt_mp0.tar: 5%|▍ | 410M/8.31G [00:02<00:47, 180MB/s]
Downloading best_ckpt_mp0.tar: 5%|▌ | 430M/8.31G [00:02<00:47, 180MB/s]
Downloading best_ckpt_mp0.tar: 5%|▌ | 450M/8.31G [00:03<00:47, 179MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 470M/8.31G [00:03<00:48, 175MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 490M/8.31G [00:03<00:48, 172MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 510M/8.31G [00:03<00:49, 170MB/s]
Downloading best_ckpt_mp0.tar: 6%|▌ | 530M/8.31G [00:03<00:50, 166MB/s]
Downloading best_ckpt_mp0.tar: 6%|▋ | 550M/8.31G [00:03<00:50, 165MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 570M/8.31G [00:03<00:49, 167MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 590M/8.31G [00:03<00:49, 167MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 610M/8.31G [00:04<00:49, 167MB/s]
Downloading best_ckpt_mp0.tar: 7%|▋ | 630M/8.31G [00:04<00:49, 166MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 650M/8.31G [00:04<00:49, 165MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 670M/8.31G [00:04<00:49, 166MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 690M/8.31G [00:04<00:49, 167MB/s]
Downloading best_ckpt_mp0.tar: 8%|▊ | 710M/8.31G [00:04<00:49, 166MB/s]
Downloading best_ckpt_mp0.tar: 9%|▊ | 730M/8.31G [00:04<00:49, 166MB/s]
Downloading best_ckpt_mp0.tar: 9%|▉ | 750M/8.31G [00:04<00:48, 167MB/s]
Downloading best_ckpt_mp0.tar: 9%|▉ | 770M/8.31G [00:05<00:49, 164MB/s]
Downloading best_ckpt_mp0.tar: 9%|▉ | 790M/8.31G [00:05<00:49, 164MB/s]
Downloading best_ckpt_mp0.tar: 10%|▉ | 810M/8.31G [00:05<00:49, 163MB/s]
Downloading best_ckpt_mp0.tar: 10%|▉ | 830M/8.31G [00:05<00:49, 163MB/s]
Downloading best_ckpt_mp0.tar: 10%|▉ | 850M/8.31G [00:05<00:49, 163MB/s]
Downloading best_ckpt_mp0.tar: 10%|█ | 870M/8.31G [00:05<00:49, 161MB/s]
Downloading best_ckpt_mp0.tar: 10%|█ | 890M/8.31G [00:05<00:48, 164MB/s]
Downloading best_ckpt_mp0.tar: 11%|█ | 910M/8.31G [00:05<00:47, 166MB/s]
Downloading best_ckpt_mp0.tar: 11%|█ | 930M/8.31G [00:06<00:46, 169MB/s]
Downloading best_ckpt_mp0.tar: 11%|█ | 950M/8.31G [00:06<00:46, 172MB/s]
Downloading best_ckpt_mp0.tar: 11%|█▏ | 970M/8.31G [00:06<00:46, 170MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 990M/8.31G [00:06<00:47, 167MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 0.99G/8.31G [00:06<00:46, 170MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 1.01G/8.31G [00:06<00:45, 172MB/s]
Downloading best_ckpt_mp0.tar: 12%|█▏ | 1.03G/8.31G [00:06<00:44, 174MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.04G/8.31G [00:06<00:44, 174MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.06G/8.31G [00:07<00:44, 174MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.08G/8.31G [00:07<00:44, 173MB/s]
Downloading best_ckpt_mp0.tar: 13%|█▎ | 1.10G/8.31G [00:07<00:44, 174MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▎ | 1.12G/8.31G [00:07<00:43, 175MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.14G/8.31G [00:07<00:43, 176MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.16G/8.31G [00:07<00:43, 178MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.18G/8.31G [00:07<00:42, 178MB/s]
Downloading best_ckpt_mp0.tar: 14%|█▍ | 1.20G/8.31G [00:07<00:42, 178MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▍ | 1.22G/8.31G [00:07<00:42, 178MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▍ | 1.24G/8.31G [00:08<00:42, 179MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▌ | 1.26G/8.31G [00:08<00:42, 178MB/s]
Downloading best_ckpt_mp0.tar: 15%|█▌ | 1.28G/8.31G [00:08<00:42, 178MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▌ | 1.30G/8.31G [00:08<00:43, 175MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▌ | 1.32G/8.31G [00:08<00:43, 173MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▌ | 1.34G/8.31G [00:08<00:42, 174MB/s]
Downloading best_ckpt_mp0.tar: 16%|█▋ | 1.36G/8.31G [00:08<00:42, 174MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.38G/8.31G [00:08<00:42, 174MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.40G/8.31G [00:09<00:42, 175MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.42G/8.31G [00:09<00:42, 176MB/s]
Downloading best_ckpt_mp0.tar: 17%|█▋ | 1.44G/8.31G [00:09<00:41, 177MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.46G/8.31G [00:09<00:41, 179MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.47G/8.31G [00:09<00:40, 180MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.49G/8.31G [00:09<00:40, 181MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.51G/8.31G [00:09<00:39, 183MB/s]
Downloading best_ckpt_mp0.tar: 18%|█▊ | 1.53G/8.31G [00:09<00:39, 184MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▊ | 1.55G/8.31G [00:09<00:39, 185MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▉ | 1.57G/8.31G [00:10<00:39, 185MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▉ | 1.59G/8.31G [00:10<00:39, 185MB/s]
Downloading best_ckpt_mp0.tar: 19%|█▉ | 1.61G/8.31G [00:10<00:45, 159MB/s]
Downloading best_ckpt_mp0.tar: 20%|█▉ | 1.63G/8.31G [00:10<00:44, 162MB/s]
Downloading best_ckpt_mp0.tar: 20%|█▉ | 1.65G/8.31G [00:10<00:55, 129MB/s]
Downloading best_ckpt_mp0.tar: 20%|██ | 1.67G/8.31G [00:10<00:58, 123MB/s]
Downloading best_ckpt_mp0.tar: 20%|██ | 1.69G/8.31G [00:11<00:53, 134MB/s]
Downloading best_ckpt_mp0.tar: 21%|██ | 1.71G/8.31G [00:11<00:49, 144MB/s]
Downloading best_ckpt_mp0.tar: 21%|██ | 1.73G/8.31G [00:11<00:53, 131MB/s]
Downloading best_ckpt_mp0.tar: 21%|██ | 1.75G/8.31G [00:11<00:50, 141MB/s]
Downloading best_ckpt_mp0.tar: 21%|██▏ | 1.77G/8.31G [00:11<01:08, 103MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.79G/8.31G [00:11<00:59, 117MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.81G/8.31G [00:12<01:00, 116MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.83G/8.31G [00:12<01:09, 100MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.85G/8.31G [00:12<01:00, 115MB/s]
Downloading best_ckpt_mp0.tar: 22%|██▏ | 1.87G/8.31G [00:12<00:54, 127MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.88G/8.31G [00:12<01:07, 102MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.90G/8.31G [00:13<01:05, 105MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.92G/8.31G [00:13<01:12, 95.2MB/s]
Downloading best_ckpt_mp0.tar: 23%|██▎ | 1.94G/8.31G [00:13<01:07, 102MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▎ | 1.96G/8.31G [00:13<00:59, 114MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▍ | 1.98G/8.31G [00:13<00:58, 117MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▍ | 2.00G/8.31G [00:14<00:58, 116MB/s]
Downloading best_ckpt_mp0.tar: 24%|██▍ | 2.02G/8.31G [00:14<00:53, 127MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▍ | 2.04G/8.31G [00:14<00:57, 116MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▍ | 2.06G/8.31G [00:14<01:01, 110MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▌ | 2.08G/8.31G [00:14<01:02, 106MB/s]
Downloading best_ckpt_mp0.tar: 25%|██▌ | 2.10G/8.31G [00:15<01:07, 99.3MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.12G/8.31G [00:15<00:58, 113MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.14G/8.31G [00:15<01:01, 108MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.16G/8.31G [00:15<00:54, 120MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▌ | 2.18G/8.31G [00:15<00:49, 132MB/s]
Downloading best_ckpt_mp0.tar: 26%|██▋ | 2.20G/8.31G [00:15<00:46, 143MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.22G/8.31G [00:15<00:42, 155MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.24G/8.31G [00:15<00:40, 163MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.26G/8.31G [00:16<00:38, 169MB/s]
Downloading best_ckpt_mp0.tar: 27%|██▋ | 2.28G/8.31G [00:16<00:37, 173MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.29G/8.31G [00:16<00:36, 176MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.31G/8.31G [00:16<00:36, 178MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.33G/8.31G [00:16<00:35, 179MB/s]
Downloading best_ckpt_mp0.tar: 28%|██▊ | 2.35G/8.31G [00:16<00:36, 177MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▊ | 2.37G/8.31G [00:16<00:35, 177MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.39G/8.31G [00:16<00:36, 176MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.41G/8.31G [00:17<00:35, 177MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.43G/8.31G [00:17<00:35, 177MB/s]
Downloading best_ckpt_mp0.tar: 29%|██▉ | 2.45G/8.31G [00:17<00:35, 175MB/s]
Downloading best_ckpt_mp0.tar: 30%|██▉ | 2.47G/8.31G [00:17<00:36, 173MB/s]
Downloading best_ckpt_mp0.tar: 30%|██▉ | 2.49G/8.31G [00:17<00:35, 176MB/s]
Downloading best_ckpt_mp0.tar: 30%|███ | 2.51G/8.31G [00:17<00:34, 180MB/s]
Downloading best_ckpt_mp0.tar: 30%|███ | 2.53G/8.31G [00:17<00:34, 181MB/s]
Downloading best_ckpt_mp0.tar: 31%|███ | 2.55G/8.31G [00:17<00:34, 182MB/s]
Downloading best_ckpt_mp0.tar: 31%|███ | 2.57G/8.31G [00:17<00:33, 182MB/s]
Downloading best_ckpt_mp0.tar: 31%|███ | 2.59G/8.31G [00:18<00:33, 183MB/s]
Downloading best_ckpt_mp0.tar: 31%|███▏ | 2.61G/8.31G [00:18<00:33, 183MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.63G/8.31G [00:18<00:33, 181MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.65G/8.31G [00:18<00:33, 181MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.67G/8.31G [00:18<00:33, 182MB/s]
Downloading best_ckpt_mp0.tar: 32%|███▏ | 2.69G/8.31G [00:18<00:33, 182MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.71G/8.31G [00:18<00:32, 182MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.72G/8.31G [00:18<00:33, 181MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.74G/8.31G [00:19<00:33, 180MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.76G/8.31G [00:19<00:33, 179MB/s]
Downloading best_ckpt_mp0.tar: 33%|███▎ | 2.78G/8.31G [00:19<00:33, 180MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▎ | 2.80G/8.31G [00:19<00:32, 180MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▍ | 2.82G/8.31G [00:19<00:33, 178MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▍ | 2.84G/8.31G [00:19<00:33, 176MB/s]
Downloading best_ckpt_mp0.tar: 34%|███▍ | 2.86G/8.31G [00:19<00:33, 174MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▍ | 2.88G/8.31G [00:19<00:33, 172MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▍ | 2.90G/8.31G [00:19<00:33, 171MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▌ | 2.92G/8.31G [00:20<00:34, 168MB/s]
Downloading best_ckpt_mp0.tar: 35%|███▌ | 2.94G/8.31G [00:20<00:34, 168MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▌ | 2.96G/8.31G [00:20<00:34, 167MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▌ | 2.98G/8.31G [00:20<00:34, 166MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▌ | 3.00G/8.31G [00:20<00:34, 166MB/s]
Downloading best_ckpt_mp0.tar: 36%|███▋ | 3.02G/8.31G [00:20<00:34, 165MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.04G/8.31G [00:20<00:34, 164MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.06G/8.31G [00:21<00:33, 167MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.08G/8.31G [00:21<00:33, 168MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.10G/8.31G [00:21<00:33, 166MB/s]
Downloading best_ckpt_mp0.tar: 37%|███▋ | 3.12G/8.31G [00:21<00:33, 165MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.13G/8.31G [00:21<00:33, 166MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.15G/8.31G [00:21<00:33, 168MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.17G/8.31G [00:21<00:32, 169MB/s]
Downloading best_ckpt_mp0.tar: 38%|███▊ | 3.19G/8.31G [00:21<00:32, 170MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▊ | 3.21G/8.31G [00:22<00:32, 171MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.23G/8.31G [00:22<00:31, 171MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.25G/8.31G [00:22<00:31, 171MB/s]
Downloading best_ckpt_mp0.tar: 39%|███▉ | 3.27G/8.31G [00:22<00:32, 168MB/s]
Downloading best_ckpt_mp0.tar: 40%|███▉ | 3.29G/8.31G [00:22<00:31, 169MB/s]
Downloading best_ckpt_mp0.tar: 40%|███▉ | 3.31G/8.31G [00:22<00:32, 167MB/s]
Downloading best_ckpt_mp0.tar: 40%|████ | 3.33G/8.31G [00:22<00:31, 167MB/s]
Downloading best_ckpt_mp0.tar: 40%|████ | 3.35G/8.31G [00:22<00:32, 166MB/s]
Downloading best_ckpt_mp0.tar: 41%|████ | 3.37G/8.31G [00:23<00:31, 168MB/s]
Downloading best_ckpt_mp0.tar: 41%|████ | 3.39G/8.31G [00:23<00:31, 166MB/s]
Downloading best_ckpt_mp0.tar: 41%|████ | 3.41G/8.31G [00:23<00:31, 169MB/s]
Downloading best_ckpt_mp0.tar: 41%|████▏ | 3.43G/8.31G [00:23<00:30, 171MB/s]
Downloading best_ckpt_mp0.tar: 41%|████▏ | 3.45G/8.31G [00:23<00:30, 172MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.47G/8.31G [00:23<00:29, 174MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.49G/8.31G [00:23<00:29, 176MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.51G/8.31G [00:23<00:30, 169MB/s]
Downloading best_ckpt_mp0.tar: 42%|████▏ | 3.53G/8.31G [00:23<00:30, 167MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.54G/8.31G [00:24<00:30, 166MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.56G/8.31G [00:24<00:30, 169MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.58G/8.31G [00:24<00:29, 170MB/s]
Downloading best_ckpt_mp0.tar: 43%|████▎ | 3.60G/8.31G [00:24<00:30, 168MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▎ | 3.62G/8.31G [00:24<00:28, 178MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▍ | 3.64G/8.31G [00:24<00:27, 181MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▍ | 3.66G/8.31G [00:24<00:27, 183MB/s]
Downloading best_ckpt_mp0.tar: 44%|████▍ | 3.68G/8.31G [00:24<00:27, 183MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▍ | 3.70G/8.31G [00:25<00:27, 183MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▍ | 3.72G/8.31G [00:25<00:26, 183MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▌ | 3.74G/8.31G [00:25<00:26, 183MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▌ | 3.76G/8.31G [00:25<00:26, 181MB/s]
Downloading best_ckpt_mp0.tar: 45%|████▌ | 3.78G/8.31G [00:25<00:27, 177MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▌ | 3.80G/8.31G [00:25<00:37, 128MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▌ | 3.82G/8.31G [00:25<00:35, 138MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▌ | 3.84G/8.31G [00:26<00:37, 129MB/s]
Downloading best_ckpt_mp0.tar: 46%|████▋ | 3.86G/8.31G [00:26<00:36, 133MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.88G/8.31G [00:26<00:48, 97.9MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.90G/8.31G [00:26<00:49, 95.2MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.92G/8.31G [00:26<00:45, 103MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.94G/8.31G [00:27<00:59, 78.9MB/s]
Downloading best_ckpt_mp0.tar: 47%|████▋ | 3.95G/8.31G [00:27<01:02, 75.0MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.96G/8.31G [00:27<00:51, 89.8MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.97G/8.31G [00:27<00:50, 92.1MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.98G/8.31G [00:27<00:54, 85.4MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 3.99G/8.31G [00:28<00:58, 79.1MB/s]
Downloading best_ckpt_mp0.tar: 48%|████▊ | 4.01G/8.31G [00:28<00:53, 85.8MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▊ | 4.03G/8.31G [00:28<00:48, 95.6MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.05G/8.31G [00:28<00:42, 107MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.07G/8.31G [00:28<00:41, 109MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.09G/8.31G [00:28<00:37, 121MB/s]
Downloading best_ckpt_mp0.tar: 49%|████▉ | 4.11G/8.31G [00:29<00:41, 110MB/s]
Downloading best_ckpt_mp0.tar: 50%|████▉ | 4.13G/8.31G [00:29<00:39, 114MB/s]
Downloading best_ckpt_mp0.tar: 50%|████▉ | 4.15G/8.31G [00:29<00:34, 128MB/s]
Downloading best_ckpt_mp0.tar: 50%|█████ | 4.17G/8.31G [00:29<00:31, 140MB/s]
Downloading best_ckpt_mp0.tar: 50%|█████ | 4.19G/8.31G [00:29<00:29, 149MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████ | 4.21G/8.31G [00:29<00:28, 157MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████ | 4.23G/8.31G [00:29<00:26, 164MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████ | 4.25G/8.31G [00:30<00:25, 168MB/s]
Downloading best_ckpt_mp0.tar: 51%|█████▏ | 4.27G/8.31G [00:30<00:25, 171MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.29G/8.31G [00:30<00:24, 175MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.31G/8.31G [00:30<00:24, 175MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.33G/8.31G [00:30<00:24, 177MB/s]
Downloading best_ckpt_mp0.tar: 52%|█████▏ | 4.35G/8.31G [00:30<00:23, 179MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.37G/8.31G [00:30<00:23, 178MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.38G/8.31G [00:30<00:23, 176MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.40G/8.31G [00:31<00:23, 176MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.42G/8.31G [00:31<00:23, 175MB/s]
Downloading best_ckpt_mp0.tar: 53%|█████▎ | 4.44G/8.31G [00:31<00:23, 176MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▎ | 4.46G/8.31G [00:31<00:23, 174MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▍ | 4.48G/8.31G [00:31<00:23, 175MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▍ | 4.50G/8.31G [00:31<00:23, 173MB/s]
Downloading best_ckpt_mp0.tar: 54%|█████▍ | 4.52G/8.31G [00:31<00:23, 172MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▍ | 4.54G/8.31G [00:31<00:22, 177MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▍ | 4.56G/8.31G [00:31<00:22, 180MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▌ | 4.58G/8.31G [00:32<00:21, 182MB/s]
Downloading best_ckpt_mp0.tar: 55%|█████▌ | 4.60G/8.31G [00:32<00:21, 183MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▌ | 4.62G/8.31G [00:32<00:21, 185MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▌ | 4.64G/8.31G [00:32<00:21, 186MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▌ | 4.66G/8.31G [00:32<00:21, 186MB/s]
Downloading best_ckpt_mp0.tar: 56%|█████▋ | 4.68G/8.31G [00:32<00:20, 186MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.70G/8.31G [00:32<00:21, 184MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.72G/8.31G [00:32<00:21, 178MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.74G/8.31G [00:33<00:21, 178MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.76G/8.31G [00:33<00:21, 177MB/s]
Downloading best_ckpt_mp0.tar: 57%|█████▋ | 4.78G/8.31G [00:33<00:21, 180MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.79G/8.31G [00:33<00:20, 182MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.81G/8.31G [00:33<00:20, 185MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.83G/8.31G [00:33<00:20, 186MB/s]
Downloading best_ckpt_mp0.tar: 58%|█████▊ | 4.85G/8.31G [00:33<00:19, 188MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▊ | 4.87G/8.31G [00:33<00:19, 188MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▉ | 4.89G/8.31G [00:33<00:19, 189MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▉ | 4.91G/8.31G [00:34<00:19, 190MB/s]
Downloading best_ckpt_mp0.tar: 59%|█████▉ | 4.93G/8.31G [00:34<00:19, 189MB/s]
Downloading best_ckpt_mp0.tar: 60%|█████▉ | 4.95G/8.31G [00:34<00:19, 189MB/s]
Downloading best_ckpt_mp0.tar: 60%|█████▉ | 4.97G/8.31G [00:34<00:18, 189MB/s]
Downloading best_ckpt_mp0.tar: 60%|██████ | 4.99G/8.31G [00:34<00:18, 188MB/s]
Downloading best_ckpt_mp0.tar: 60%|██████ | 5.01G/8.31G [00:34<00:19, 178MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.03G/8.31G [00:34<00:20, 168MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.05G/8.31G [00:34<00:21, 161MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.07G/8.31G [00:35<00:21, 163MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████ | 5.09G/8.31G [00:35<00:21, 164MB/s]
Downloading best_ckpt_mp0.tar: 61%|██████▏ | 5.11G/8.31G [00:35<00:21, 157MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.13G/8.31G [00:35<00:21, 159MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.15G/8.31G [00:35<00:21, 159MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.17G/8.31G [00:35<00:20, 161MB/s]
Downloading best_ckpt_mp0.tar: 62%|██████▏ | 5.19G/8.31G [00:35<00:20, 160MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.21G/8.31G [00:35<00:20, 160MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.22G/8.31G [00:36<00:20, 160MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.24G/8.31G [00:36<00:20, 158MB/s]
Downloading best_ckpt_mp0.tar: 63%|██████▎ | 5.26G/8.31G [00:36<00:20, 158MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▎ | 5.28G/8.31G [00:36<00:20, 160MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▍ | 5.30G/8.31G [00:36<00:20, 157MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▍ | 5.32G/8.31G [00:36<00:20, 157MB/s]
Downloading best_ckpt_mp0.tar: 64%|██████▍ | 5.34G/8.31G [00:36<00:20, 157MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▍ | 5.36G/8.31G [00:37<00:20, 157MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▍ | 5.38G/8.31G [00:37<00:20, 157MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▍ | 5.40G/8.31G [00:37<00:19, 160MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▌ | 5.42G/8.31G [00:37<00:19, 160MB/s]
Downloading best_ckpt_mp0.tar: 65%|██████▌ | 5.44G/8.31G [00:37<00:19, 157MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▌ | 5.46G/8.31G [00:37<00:19, 156MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▌ | 5.48G/8.31G [00:37<00:19, 157MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▌ | 5.50G/8.31G [00:37<00:19, 156MB/s]
Downloading best_ckpt_mp0.tar: 66%|██████▋ | 5.52G/8.31G [00:38<00:19, 156MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.54G/8.31G [00:38<00:18, 157MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.56G/8.31G [00:38<00:18, 157MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.58G/8.31G [00:38<00:18, 158MB/s]
Downloading best_ckpt_mp0.tar: 67%|██████▋ | 5.60G/8.31G [00:38<00:18, 158MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.62G/8.31G [00:38<00:18, 157MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.63G/8.31G [00:38<00:18, 158MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.65G/8.31G [00:38<00:17, 159MB/s]
Downloading best_ckpt_mp0.tar: 68%|██████▊ | 5.67G/8.31G [00:39<00:17, 158MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▊ | 5.69G/8.31G [00:39<00:17, 156MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.71G/8.31G [00:39<00:17, 157MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.73G/8.31G [00:39<00:17, 157MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.75G/8.31G [00:39<00:17, 155MB/s]
Downloading best_ckpt_mp0.tar: 69%|██████▉ | 5.77G/8.31G [00:39<00:17, 158MB/s]
Downloading best_ckpt_mp0.tar: 70%|██████▉ | 5.79G/8.31G [00:39<00:16, 160MB/s]
Downloading best_ckpt_mp0.tar: 70%|██████▉ | 5.81G/8.31G [00:40<00:16, 161MB/s]
Downloading best_ckpt_mp0.tar: 70%|███████ | 5.83G/8.31G [00:40<00:16, 161MB/s]
Downloading best_ckpt_mp0.tar: 70%|███████ | 5.85G/8.31G [00:40<00:16, 161MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████ | 5.87G/8.31G [00:40<00:16, 161MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████ | 5.89G/8.31G [00:40<00:15, 163MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████ | 5.91G/8.31G [00:40<00:15, 165MB/s]
Downloading best_ckpt_mp0.tar: 71%|███████▏ | 5.93G/8.31G [00:40<00:15, 164MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.95G/8.31G [00:40<00:15, 162MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.97G/8.31G [00:41<00:15, 165MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 5.99G/8.31G [00:41<00:14, 169MB/s]
Downloading best_ckpt_mp0.tar: 72%|███████▏ | 6.01G/8.31G [00:41<00:14, 173MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.03G/8.31G [00:41<00:13, 177MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.04G/8.31G [00:41<00:13, 176MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.06G/8.31G [00:41<00:15, 154MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.08G/8.31G [00:41<00:14, 162MB/s]
Downloading best_ckpt_mp0.tar: 73%|███████▎ | 6.10G/8.31G [00:41<00:15, 152MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▎ | 6.12G/8.31G [00:42<00:15, 154MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▍ | 6.14G/8.31G [00:42<00:14, 156MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▍ | 6.16G/8.31G [00:42<00:14, 158MB/s]
Downloading best_ckpt_mp0.tar: 74%|███████▍ | 6.18G/8.31G [00:42<00:14, 161MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▍ | 6.20G/8.31G [00:42<00:20, 108MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▍ | 6.22G/8.31G [00:42<00:18, 120MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▌ | 6.24G/8.31G [00:43<00:16, 131MB/s]
Downloading best_ckpt_mp0.tar: 75%|███████▌ | 6.26G/8.31G [00:43<00:15, 139MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▌ | 6.28G/8.31G [00:43<00:14, 147MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▌ | 6.30G/8.31G [00:43<00:14, 152MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▌ | 6.32G/8.31G [00:43<00:13, 156MB/s]
Downloading best_ckpt_mp0.tar: 76%|███████▋ | 6.34G/8.31G [00:43<00:13, 158MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.36G/8.31G [00:43<00:12, 162MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.38G/8.31G [00:43<00:12, 167MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.40G/8.31G [00:44<00:13, 155MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.42G/8.31G [00:44<00:12, 156MB/s]
Downloading best_ckpt_mp0.tar: 77%|███████▋ | 6.44G/8.31G [00:44<00:12, 158MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.46G/8.31G [00:44<00:12, 165MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.47G/8.31G [00:44<00:11, 170MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.49G/8.31G [00:44<00:11, 170MB/s]
Downloading best_ckpt_mp0.tar: 78%|███████▊ | 6.51G/8.31G [00:44<00:11, 169MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▊ | 6.53G/8.31G [00:45<00:11, 170MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▉ | 6.55G/8.31G [00:45<00:10, 173MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▉ | 6.57G/8.31G [00:45<00:10, 174MB/s]
Downloading best_ckpt_mp0.tar: 79%|███████▉ | 6.59G/8.31G [00:45<00:10, 172MB/s]
Downloading best_ckpt_mp0.tar: 80%|███████▉ | 6.61G/8.31G [00:45<00:10, 175MB/s]
Downloading best_ckpt_mp0.tar: 80%|███████▉ | 6.63G/8.31G [00:45<00:10, 177MB/s]
Downloading best_ckpt_mp0.tar: 80%|████████ | 6.65G/8.31G [00:45<00:10, 175MB/s]
Downloading best_ckpt_mp0.tar: 80%|████████ | 6.67G/8.31G [00:45<00:09, 177MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.69G/8.31G [00:45<00:09, 177MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.71G/8.31G [00:46<00:09, 178MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.73G/8.31G [00:46<00:09, 175MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████ | 6.75G/8.31G [00:46<00:09, 174MB/s]
Downloading best_ckpt_mp0.tar: 81%|████████▏ | 6.77G/8.31G [00:46<00:09, 172MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.79G/8.31G [00:46<00:09, 173MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.81G/8.31G [00:46<00:09, 173MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.83G/8.31G [00:46<00:09, 170MB/s]
Downloading best_ckpt_mp0.tar: 82%|████████▏ | 6.85G/8.31G [00:46<00:08, 175MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.87G/8.31G [00:47<00:08, 179MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.88G/8.31G [00:47<00:08, 181MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.90G/8.31G [00:47<00:08, 184MB/s]
Downloading best_ckpt_mp0.tar: 83%|████████▎ | 6.92G/8.31G [00:47<00:07, 186MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▎ | 6.94G/8.31G [00:47<00:07, 186MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▍ | 6.96G/8.31G [00:47<00:07, 186MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▍ | 6.98G/8.31G [00:47<00:07, 184MB/s]
Downloading best_ckpt_mp0.tar: 84%|████████▍ | 7.00G/8.31G [00:47<00:07, 182MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▍ | 7.02G/8.31G [00:47<00:07, 178MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▍ | 7.04G/8.31G [00:48<00:07, 178MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▍ | 7.06G/8.31G [00:48<00:07, 179MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▌ | 7.08G/8.31G [00:48<00:07, 180MB/s]
Downloading best_ckpt_mp0.tar: 85%|████████▌ | 7.10G/8.31G [00:48<00:07, 182MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▌ | 7.12G/8.31G [00:48<00:07, 182MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▌ | 7.14G/8.31G [00:48<00:06, 182MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▌ | 7.16G/8.31G [00:48<00:06, 183MB/s]
Downloading best_ckpt_mp0.tar: 86%|████████▋ | 7.18G/8.31G [00:48<00:06, 181MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.20G/8.31G [00:48<00:06, 178MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.22G/8.31G [00:49<00:06, 175MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.24G/8.31G [00:49<00:06, 176MB/s]
Downloading best_ckpt_mp0.tar: 87%|████████▋ | 7.26G/8.31G [00:49<00:06, 177MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.28G/8.31G [00:49<00:06, 179MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.29G/8.31G [00:49<00:06, 180MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.31G/8.31G [00:49<00:05, 181MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.33G/8.31G [00:49<00:05, 183MB/s]
Downloading best_ckpt_mp0.tar: 88%|████████▊ | 7.35G/8.31G [00:49<00:05, 185MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▊ | 7.37G/8.31G [00:50<00:05, 187MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▉ | 7.39G/8.31G [00:50<00:05, 187MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▉ | 7.41G/8.31G [00:50<00:05, 188MB/s]
Downloading best_ckpt_mp0.tar: 89%|████████▉ | 7.43G/8.31G [00:50<00:04, 189MB/s]
Downloading best_ckpt_mp0.tar: 90%|████████▉ | 7.45G/8.31G [00:50<00:04, 189MB/s]
Downloading best_ckpt_mp0.tar: 90%|████████▉ | 7.47G/8.31G [00:50<00:04, 186MB/s]
Downloading best_ckpt_mp0.tar: 90%|█████████ | 7.49G/8.31G [00:50<00:04, 183MB/s]
Downloading best_ckpt_mp0.tar: 90%|█████████ | 7.51G/8.31G [00:50<00:04, 181MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████ | 7.53G/8.31G [00:50<00:04, 177MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████ | 7.55G/8.31G [00:51<00:04, 177MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████ | 7.57G/8.31G [00:51<00:04, 163MB/s]
Downloading best_ckpt_mp0.tar: 91%|█████████▏| 7.59G/8.31G [00:51<00:04, 161MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.61G/8.31G [00:51<00:04, 168MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.63G/8.31G [00:51<00:04, 174MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.65G/8.31G [00:51<00:03, 179MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.67G/8.31G [00:51<00:03, 181MB/s]
Downloading best_ckpt_mp0.tar: 92%|█████████▏| 7.69G/8.31G [00:51<00:03, 180MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.71G/8.31G [00:52<00:03, 175MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.72G/8.31G [00:52<00:03, 174MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.74G/8.31G [00:52<00:03, 174MB/s]
Downloading best_ckpt_mp0.tar: 93%|█████████▎| 7.76G/8.31G [00:52<00:03, 173MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▎| 7.78G/8.31G [00:52<00:03, 176MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▍| 7.80G/8.31G [00:52<00:03, 178MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▍| 7.82G/8.31G [00:52<00:02, 183MB/s]
Downloading best_ckpt_mp0.tar: 94%|█████████▍| 7.84G/8.31G [00:52<00:02, 186MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▍| 7.86G/8.31G [00:52<00:02, 187MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▍| 7.88G/8.31G [00:53<00:02, 187MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▌| 7.90G/8.31G [00:53<00:02, 186MB/s]
Downloading best_ckpt_mp0.tar: 95%|█████████▌| 7.92G/8.31G [00:53<00:02, 185MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.94G/8.31G [00:53<00:02, 185MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.96G/8.31G [00:53<00:02, 185MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▌| 7.98G/8.31G [00:53<00:01, 182MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▋| 8.00G/8.31G [00:53<00:01, 181MB/s]
Downloading best_ckpt_mp0.tar: 96%|█████████▋| 8.02G/8.31G [00:53<00:01, 180MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.04G/8.31G [00:54<00:01, 175MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.06G/8.31G [00:54<00:01, 176MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.08G/8.31G [00:54<00:01, 178MB/s]
Downloading best_ckpt_mp0.tar: 97%|█████████▋| 8.10G/8.31G [00:54<00:01, 180MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.12G/8.31G [00:54<00:01, 175MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.13G/8.31G [00:54<00:01, 174MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.15G/8.31G [00:54<00:00, 175MB/s]
Downloading best_ckpt_mp0.tar: 98%|█████████▊| 8.17G/8.31G [00:54<00:00, 177MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▊| 8.19G/8.31G [00:54<00:00, 178MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▉| 8.21G/8.31G [00:55<00:00, 176MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▉| 8.23G/8.31G [00:55<00:00, 175MB/s]
Downloading best_ckpt_mp0.tar: 99%|█████████▉| 8.25G/8.31G [00:55<00:00, 176MB/s]
Downloading best_ckpt_mp0.tar: 100%|█████████▉| 8.27G/8.31G [00:55<00:00, 175MB/s]
Downloading best_ckpt_mp0.tar: 100%|█████████▉| 8.29G/8.31G [00:55<00:00, 181MB/s]
Downloading best_ckpt_mp0.tar: 100%|██████████| 8.31G/8.31G [00:55<00:00, 181MB/s]
Downloading best_ckpt_mp0.tar: 100%|██████████| 8.31G/8.31G [00:55<00:00, 160MB/s]
2025-05-16 00:35:02.448 | INFO | earth2studio.run:ensemble:315 - Running ensemble inference!
2025-05-16 00:35:02.449 | INFO | earth2studio.run:ensemble:323 - Inference device: cuda
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.756 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 169090127-920171
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.779 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210820017-615282
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.802 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 407591936-940269
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.825 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 190027998-577814
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.848 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 252556659-958137
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.871 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 232400432-586007
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.894 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184050412-731750
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.917 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 149106074-462399
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.940 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 196462617-1008822
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.963 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 346879212-932889
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:03.986 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 189450163-577835
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.009 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 143527711-755695
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.032 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 393705863-838502
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.054 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 275180657-890286
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.078 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 347812101-849637
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.102 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 270785488-1177846
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.126 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 350072351-1232650
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.149 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 144283406-743766
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.172 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199934883-595444
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.194 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 295659093-896559
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.217 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 179198897-973788
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.241 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 402321768-876246
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.264 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 330670600-938837
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.288 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 193737151-726758
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.312 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 246334297-805355
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.335 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 194463909-743465
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.358 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 206944850-1065961
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.381 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 0-993995
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.404 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 383286081-1212507
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.428 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 148613430-492644
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.450 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 186591756-1053680
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.473 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 381424372-852801
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.496 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 406629528-962408
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.519 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 247139652-717479
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.541 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174160271-736006
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.565 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 387322476-948106
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.588 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 176434139-1041731
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.611 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 326166249-1225442
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.634 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268038708-839499
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.657 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184782162-755841
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.679 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323061199-895080
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.702 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 451628742-968328
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.725 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 170010298-904882
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.747 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204118947-720169
Fetching GFS data: 0%| | 0/74 [00:00<?, ?it/s]
2025-05-16 00:35:04.770 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323956279-837771
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.792 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 386359113-963363
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.815 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 452597070-961302
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.837 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 274285343-895314
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.860 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 329739828-930772
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.883 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 253514796-920355
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.906 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 296555652-890544
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.929 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 226457235-723507
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.951 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174896277-746582
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.974 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 292088625-1244938
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:04.997 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 391722290-987401
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.020 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 163781343-739174
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.042 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199346060-588823
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.065 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 145318799-1019625
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.087 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 180172685-913532
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.110 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 290159183-752827
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.133 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 414179964-1179422
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.156 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 353619033-939943
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.179 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 354558976-950206
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.202 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 231827443-572989
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.225 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210217024-602993
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.248 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 225640684-816551
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.271 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204839116-753955
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.293 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 228474082-1138597
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.316 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 164520517-765194
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.339 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 404382959-913875
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.361 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 289307267-851916
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.385 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 165935718-1138677
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.408 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268878207-724790
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
2025-05-16 00:35:05.431 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 249098202-1262404
Fetching GFS data: 0%| | 0/74 [00:01<?, ?it/s]
Fetching GFS data: 1%|▏ | 1/74 [00:01<02:03, 1.70s/it]
Fetching GFS data: 100%|██████████| 74/74 [00:01<00:00, 43.57it/s]
2025-05-16 00:35:05.782 | SUCCESS | earth2studio.run:ensemble:345 - Fetched data from GFS
2025-05-16 00:35:05.789 | WARNING | earth2studio.io.zarr:add_array:192 - Datetime64 not supported in zarr 3.0, converting to int64 nanoseconds since epoch
2025-05-16 00:35:05.795 | WARNING | earth2studio.io.zarr:add_array:198 - Timedelta64 not supported in zarr 3.0, converting to int64 nanoseconds since epoch
2025-05-16 00:35:05.815 | INFO | earth2studio.run:ensemble:373 - Starting 2 Member Ensemble Inference with 2 number of batches.
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
2025-05-16 00:35:05.830 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 148091792-1031496
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:05.857 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 392065452-969982
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:05.884 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210217024-602993
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:05.908 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 300299872-884864
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:05.931 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 387152087-1211521
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:05.956 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 193097116-578707
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:05.979 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 330670600-938837
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.004 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 257413354-553231
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.027 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 459841403-963517
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.051 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 230071239-818111
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.074 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 414179964-1179422
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.098 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 354558976-950206
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.123 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 251417951-723202
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.147 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 399434135-840126
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.170 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 200082859-1168749
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.194 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 190027998-577814
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.217 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 167362967-767433
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.240 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 232576858-1266241
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.265 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 329654990-1225009
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.289 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 359046224-949853
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.313 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 189450163-577835
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.336 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 206944850-1065961
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.359 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 211043451-1192232
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.383 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 148724824-1026834
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.408 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 232918171-1262978
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.432 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 296555652-890544
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.457 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 236647942-590553
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.481 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 208052937-721817
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.505 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199346060-588823
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.529 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 191094271-1176950
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.553 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 165935718-1138677
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.578 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 394736180-951384
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.601 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 258516328-918758
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.624 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 404382959-913875
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.647 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 182403895-973883
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.671 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 180172685-913532
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.694 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 350547115-929875
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.717 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 179476202-1048997
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.741 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 150405231-492889
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.764 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 148613430-492644
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.786 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 194463909-743465
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.810 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 145318799-1019625
Fetching GFS data: 0%| | 0/296 [00:00<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:00<?, ?it/s]
2025-05-16 00:35:06.833 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 408062467-879185
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.857 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 176434139-1041731
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.880 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 251230645-803982
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.903 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 179198897-973788
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.927 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 327423370-840295
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.950 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 232267599-726560
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.973 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 246334297-805355
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:06.997 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 278865492-888548
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.020 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 146927534-759351
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.044 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 197360802-728078
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.068 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 169090127-920171
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.092 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 387322476-948106
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.115 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 171310346-917594
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.138 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 210882735-1186600
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.162 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 193241168-579502
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.185 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 334970306-938022
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.209 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204839116-753955
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.232 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 458443991-962968
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.255 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 186591756-1053680
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.279 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 183507493-913609
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.302 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 178183898-738189
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.325 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 237104567-587286
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.349 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 236071718-576224
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.373 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 166625127-737840
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.396 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 393767669-968511
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.419 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 397639332-840040
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.442 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 182534428-973065
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.465 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 271656637-839000
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.489 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 189280019-753310
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.513 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 187292486-732506
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.536 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 188158210-757568
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.559 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 457464510-979481
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.582 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 275180657-890286
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.605 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 326532594-890776
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.629 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 167248172-739678
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.653 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 176832054-736857
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.676 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 252556659-958137
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.699 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 226457235-723507
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.722 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 204088265-596882
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.746 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 237907755-575171
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.769 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 294691465-856457
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.792 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 196462617-1008822
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:01<?, ?it/s]
2025-05-16 00:35:07.815 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 197458039-726350
Fetching GFS data: 0%| | 0/296 [00:01<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.838 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 413569354-967478
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.862 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 406073657-506593
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.884 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 214171943-607657
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.907 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 180472580-1170586
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.930 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 152534337-466715
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.953 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 295659093-896559
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:07.977 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 215007021-618021
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.000 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 198184389-747202
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.023 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 354932182-856465
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.046 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 231827443-572989
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.069 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 225640684-816551
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.093 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 193820670-578278
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.116 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 401150630-841557
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.139 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 215757651-606283
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.162 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 238482926-588964
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.185 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 165594251-738958
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.208 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 172072425-918801
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.231 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 351476990-852387
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.255 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 281281791-898336
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.278 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 413380602-945786
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.301 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 167747263-1134797
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.325 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 151904457-465264
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.348 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 361723180-950913
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.372 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 410138186-925503
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.395 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 402321768-876246
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.419 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 199934883-595444
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.441 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 199460585-743054
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.465 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 397402829-996456
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.489 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 386359113-963363
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.512 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 177186467-735476
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.535 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 353761167-1225423
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.558 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 409589953-513282
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.581 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 282180127-893274
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.605 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 301084617-899856
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.628 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 247139652-717479
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.651 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 230544483-732296
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.674 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 412411490-969112
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.697 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 228474082-1138597
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.722 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 0-1031836
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.745 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 293912488-745515
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.768 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 216363934-618291
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.791 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 203992655-596596
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.814 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 383286081-1212507
Fetching GFS data: 0%| | 0/296 [00:02<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:02<?, ?it/s]
2025-05-16 00:35:08.841 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 297291574-754222
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:08.866 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 177568911-747761
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:08.891 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 253514796-920355
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:08.914 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 456116956-963873
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:08.938 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 208928508-761990
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:08.961 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 253388728-1269329
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:08.985 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 302819301-896743
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.010 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 205351099-596883
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.033 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 145304873-758046
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.056 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 272495637-727089
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.080 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 200175442-1167472
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.104 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 390660597-1219295
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.128 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 147098312-1020507
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.153 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 390243670-963082
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.177 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 414536832-944207
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.200 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 455143487-973469
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.223 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 204760966-590133
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.246 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 260003950-922575
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.270 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 169411507-1139754
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.294 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 252718154-803297
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.319 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 198088880-741667
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.344 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 249098202-1262404
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.369 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 147055134-746039
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.393 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 336687879-934577
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.418 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 279542923-898410
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.442 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268878207-724790
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.465 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 395625686-986980
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.489 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 274408443-1223885
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.513 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 360780804-942376
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.536 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 188547657-732362
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.564 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 357218775-1228059
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.591 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 0-998436
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.616 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 173902504-907905
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.639 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 393035434-953486
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.663 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 189840662-1179194
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.688 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 204118947-720169
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.713 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 350072351-1232650
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.739 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 151411717-492740
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.764 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 179108822-1168912
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.789 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 170010298-904882
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.814 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 421300034-1181973
Fetching GFS data: 0%| | 0/296 [00:03<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:03<?, ?it/s]
2025-05-16 00:35:09.838 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 420029701-1181204
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:09.862 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 410028724-962181
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:09.885 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 209414924-720687
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:09.909 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 330695860-846471
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:09.933 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 230889350-725457
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:09.958 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 270785488-1177846
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:09.982 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 357316538-939003
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.005 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 152040961-493376
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.028 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 337622456-936189
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.052 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 359996077-949678
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.077 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 187427126-731084
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.102 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 208774754-755459
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.126 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 232400432-586007
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.149 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 177921943-752161
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.172 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 172983015-919489
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.195 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 194932669-578572
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.219 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 193675823-578990
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.242 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 326166249-1225442
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.266 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 198733171-727414
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.289 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 332945430-1359233
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.313 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 188024992-754829
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.337 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184782162-755841
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.361 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 183650695-972676
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.384 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 303716044-887380
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.407 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 275795645-726593
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.430 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 353190489-858785
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.455 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 452597070-961302
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.479 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 193737151-726758
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.501 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 333255379-930566
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.524 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 411298483-922437
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.547 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 257599990-916338
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.569 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 292088625-1244938
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.594 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 295820475-1242797
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.618 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 299224343-1245614
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.642 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 387075654-860051
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.665 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 451628742-968328
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.687 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 189979250-1176980
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.710 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 184623371-911795
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.733 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 335908328-940530
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.757 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 290159183-752827
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.780 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 214779600-623304
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.804 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 252034627-722839
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:04<?, ?it/s]
2025-05-16 00:35:10.827 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 407591936-940269
Fetching GFS data: 0%| | 0/296 [00:04<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.850 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 184050412-731750
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.874 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 256868469-544885
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.897 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 178922087-749074
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.919 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 183377778-912918
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.942 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323061199-895080
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.965 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 329116923-847018
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:10.988 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 254001564-1267820
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.013 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174896277-746582
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.036 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 301984473-889315
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.059 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 323956279-837771
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.082 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 358255541-950771
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.105 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 194353089-579580
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.127 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 277722016-1226189
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.151 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 0-993995
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.174 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 329739828-930772
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.197 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 346879212-932889
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.220 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 410990905-942963
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.242 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 168789447-980831
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.266 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 274954839-840806
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.290 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 255488233-1268872
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.314 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 149106074-462399
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.337 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 172227940-905153
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.360 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 250614479-803472
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.383 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 385279214-852759
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.406 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 329799511-896349
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.430 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 273976632-728286
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.452 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 406629528-962408
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.476 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 417602460-1179045
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.499 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 277968948-896544
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.523 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 280441333-889661
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.546 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 164520517-765194
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.569 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 347812101-849637
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.592 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 259087914-916036
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.615 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 296442783-848791
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.638 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 273134252-842380
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.661 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 328218119-898804
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.685 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 210820017-615282
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.708 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 407776106-914088
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.731 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 0-1002356
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.754 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 353999639-932543
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.777 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 167987850-767526
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.800 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 234294213-1264469
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:05<?, ?it/s]
2025-05-16 00:35:11.823 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 388958699-1221367
Fetching GFS data: 0%| | 0/296 [00:05<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.847 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 174160271-736006
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.871 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 299407315-892557
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.894 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 236529889-574678
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.917 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 393705863-838502
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.939 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 289307267-851916
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.962 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 334185945-934655
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:11.985 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 147686885-749156
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.008 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 388773222-859658
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.031 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 352251507-938982
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.055 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 297490896-1247432
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.078 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 391206752-950631
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.101 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 150898120-463977
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.124 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 144283406-743766
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.146 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 381424372-852801
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.170 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 203498423-589842
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.193 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 229726800-817683
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.216 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 231450583-817016
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.240 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 212236463-1187155
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.264 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 166333209-766399
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.287 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 172991226-911247
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.310 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 214401793-605228
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.333 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 331362340-1232012
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.357 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 353619033-939943
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.381 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 293067730-844758
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.404 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 399119260-992978
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.428 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 210135611-756844
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.451 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 295547922-758800
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.475 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 355472229-1239204
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.499 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 146298066-757068
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.522 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 253521451-719382
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.545 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 274285343-895314
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.569 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 143527711-755695
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.593 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 391722290-987401
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.616 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 201444244-1166535
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.639 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 146062919-744726
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.661 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 275899947-1309015
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.688 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 163781343-739174
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.712 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/06/atmos/gfs.t06z.pgrb2.0p25.f000 208203900-724608
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.736 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/18/atmos/gfs.t18z.pgrb2.0p25.f000 203402796-589859
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.759 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20240101/00/atmos/gfs.t00z.pgrb2.0p25.f000 268038708-839499
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
2025-05-16 00:35:12.783 | DEBUG | earth2studio.data.gfs:fetch_array:353 - Fetching GFS grib file: noaa-gfs-bdp-pds/gfs.20231231/12/atmos/gfs.t12z.pgrb2.0p25.f000 458864763-976640
Fetching GFS data: 0%| | 0/296 [00:06<?, ?it/s]
Total Ensemble Batches: 0%| | 0/2 [00:06<?, ?it/s]
Fetching GFS data: 0%| | 1/296 [00:06<34:18, 6.98s/it]
Fetching GFS data: 100%|██████████| 296/296 [00:06<00:00, 42.42it/s]
Running batch 0 inference: 0%| | 0/5 [00:00<?, ?it/s]
Running batch 0 inference: 20%|██ | 1/5 [00:00<00:00, 4.64it/s]
Running batch 0 inference: 40%|████ | 2/5 [00:01<00:02, 1.15it/s]
Running batch 0 inference: 60%|██████ | 3/5 [00:02<00:02, 1.07s/it]
Running batch 0 inference: 80%|████████ | 4/5 [00:04<00:01, 1.15s/it]
Running batch 0 inference: 100%|██████████| 5/5 [00:05<00:00, 1.22s/it]
Total Ensemble Batches: 50%|█████ | 1/2 [00:25<00:25, 25.91s/it]
Running batch 1 inference: 0%| | 0/5 [00:00<?, ?it/s]
Running batch 1 inference: 20%|██ | 1/5 [00:00<00:01, 3.81it/s]
Running batch 1 inference: 40%|████ | 2/5 [00:01<00:02, 1.15it/s]
Running batch 1 inference: 60%|██████ | 3/5 [00:02<00:02, 1.08s/it]
Running batch 1 inference: 80%|████████ | 4/5 [00:04<00:01, 1.18s/it]
Running batch 1 inference: 100%|██████████| 5/5 [00:05<00:00, 1.22s/it]
Total Ensemble Batches: 100%|██████████| 2/2 [00:31<00:00, 13.90s/it]
Total Ensemble Batches: 100%|██████████| 2/2 [00:31<00:00, 15.70s/it]
2025-05-16 00:35:37.221 | SUCCESS | earth2studio.run:ensemble:423 - Inference complete
/
├── ensemble (2,) int64
├── lat (721,) float64
├── lead_time (5,) int64
├── lon (1440,) float64
├── time (1,) int64
├── u10m (2, 1, 5, 721, 1440) float32
└── v10m (2, 1, 5, 721, 1440) float32
Post Processing#
The result of the workflow is two zarr stores with the ensemble data for the respective checkpoints used. The rest of the example is focused on some basic post processing to visualize the results.
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
import numpy as np
import xarray as xr
lead_time = 4
plot_date = start_date + timedelta(hours=int(6 * lead_time))
# Load data from both zarr stores
ds0 = xr.open_zarr("outputs/11_hens_0.zarr")
ds1 = xr.open_zarr("outputs/11_hens_1.zarr")
# Combine the datasets
ds = xr.concat([ds0, ds1], dim="ensemble")
# Calculate wind speed magnitude
wind_speed = np.sqrt(ds.u10m**2 + ds.v10m**2)
# Get mean and std of 4th timestep across ensemble
mean_wind = wind_speed.isel(time=0, lead_time=lead_time).mean(dim="ensemble")
std_wind = wind_speed.isel(time=0, lead_time=lead_time).std(dim="ensemble")
# Create figure with two subplots
fig, (ax1, ax2) = plt.subplots(
1, 2, figsize=(15, 4), subplot_kw={"projection": ccrs.PlateCarree()}
)
# Plot mean
p1 = ax1.contourf(
mean_wind.coords["lon"],
mean_wind.coords["lat"],
mean_wind,
levels=15,
transform=ccrs.PlateCarree(),
cmap="nipy_spectral",
)
ax1.coastlines()
ax1.set_title(f'Mean Wind Speed\n{plot_date.strftime("%Y-%m-%d %H:%M UTC")}')
fig.colorbar(p1, ax=ax1, label="m/s")
# Plot standard deviation
p2 = ax2.contourf(
std_wind.coords["lon"],
std_wind.coords["lat"],
std_wind,
levels=15,
transform=ccrs.PlateCarree(),
cmap="viridis",
)
ax2.coastlines()
ax2.set_title(
f'Wind Speed Standard Deviation\n{plot_date.strftime("%Y-%m-%d %H:%M UTC")}'
)
fig.colorbar(p2, ax=ax2, label="m/s")
plt.tight_layout()
# Save the figure
plt.savefig(f"outputs/11_hens_step_{plot_date.strftime('%Y_%m_%d')}.jpg")
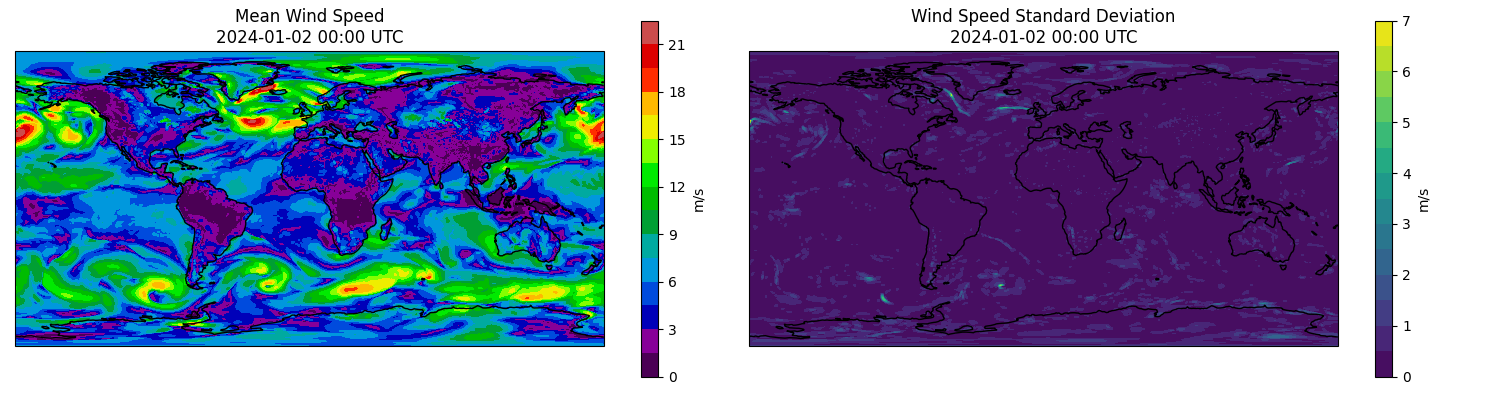
Total running time of the script: (4 minutes 35.283 seconds)