Note
Go to the end to download the full example code.
Running DLESyM Inference#
Basic deterministic inference workflow for the DLESyM model.
This example will demonstrate how to run a simple inference workflow with the DLESyM model, which differs from other prognostic models in earth2studio. DLESyM performs global earth system modeling, including atmosphere and ocean components which evolve on different timescales (different temporal resolution). Internally, the model uses a HEALPix nside=64 (approximately 1 degree) resolution grid for the physical variables of interest. The model also uses some derived input variables which are not provided by standard data sources but can be computed from the standard variables.
In this example you will learn:
How to instantiate the DLESyM model
How to use the model API to generate a forecast
How to use the output selection and regridding methods to select appropriate data
How to use the DLESyMLatLon model with earth2studio workflows
Set Up#
The first step is fetching appropriate input data for the model. The ERA5 data sources in earth2studio provide data on the lat/lon grid, so have two options:
Use the
earth2studio.models.px.DLESyMLatLon
model. This version of DLESyM accepts inputs on the lat/lon grid and regrids them to the HEALPix grid internally, before returning the output regridded back to the lat/lon grid. This is the recommended approach for most users as it can be used directly with earth2studio data sources and workflows, since it performs regridding and pre-processing internally.Use the
earth2studio.models.px.DLESyM
model, and handle the regridding of input lat/lon data ourselves. Since the model uses some derived variables which are not provided by the data source, we would also need to prepare these derived variables ourselves.
Let’s load both of these models and inspect the expected input coordinates for each. Also note the input and output variable set for each model.
import os
os.makedirs("outputs", exist_ok=True)
from dotenv import load_dotenv
load_dotenv() # TODO: make common example prep function
import numpy as np
import torch
from earth2studio.data import ARCO
from earth2studio.data.utils import fetch_data
from earth2studio.models.px.dlesym import DLESyM, DLESyMLatLon
device = "cpu" if not torch.cuda.is_available() else "cuda"
# Create the data source
data = ARCO()
# Load the default model package which downloads the check point from NGC
# We will instantiate both versions of the model to demonstrate usage of each.
package = DLESyMLatLon.load_default_package()
model_ll = DLESyMLatLon.load_model(package).to(device)
package = DLESyM.load_default_package()
model_hpx = DLESyM.load_model(package).to(device)
in_coords_ll = model_ll.input_coords()
in_coords_hpx = model_hpx.input_coords()
print(
"DLESyM LatLon input coord shapes: ",
[(k, v.shape) for k, v in in_coords_ll.items()],
)
print(
"DLESyM HPX input coord shapes: ", [(k, v.shape) for k, v in in_coords_hpx.items()]
)
print("Lat-lon input variable names: ", in_coords_ll["variable"])
print(
"Lat-lon output variable names: ", model_ll.output_coords(in_coords_ll)["variable"]
)
print("HEALPix input variable names: ", in_coords_hpx["variable"])
print(
"HEALPix output variable names: ",
model_hpx.output_coords(in_coords_hpx)["variable"],
)
Downloading config.yaml: 0%| | 0.00/1.36k [00:00<?, ?B/s]
Downloading config.yaml: 100%|██████████| 1.36k/1.36k [00:00<00:00, 1.36MB/s]
Downloading atmos_model_0.mdlus: 0%| | 0.00/37.6M [00:00<?, ?B/s]
Downloading atmos_model_0.mdlus: 4%|▍ | 1.42M/37.6M [00:00<00:02, 14.9MB/s]
Downloading atmos_model_0.mdlus: 52%|█████▏ | 19.7M/37.6M [00:00<00:00, 119MB/s]
Downloading atmos_model_0.mdlus: 100%|██████████| 37.6M/37.6M [00:00<00:00, 138MB/s]
Downloading ocean_model_0.mdlus: 0%| | 0.00/2.97M [00:00<?, ?B/s]
Downloading ocean_model_0.mdlus: 71%|███████▏ | 2.12M/2.97M [00:00<00:00, 21.3MB/s]
Downloading ocean_model_0.mdlus: 100%|██████████| 2.97M/2.97M [00:00<00:00, 23.8MB/s]
Warning, cannot find healpixpad module
Downloading hpx_lat.npy: 0%| | 0.00/384k [00:00<?, ?B/s]
Downloading hpx_lat.npy: 100%|██████████| 384k/384k [00:00<00:00, 15.3MB/s]
Downloading hpx_lon.npy: 0%| | 0.00/384k [00:00<?, ?B/s]
Downloading hpx_lon.npy: 100%|██████████| 384k/384k [00:00<00:00, 36.5MB/s]
Downloading land_sea_mask.npy: 0%| | 0.00/192k [00:00<?, ?B/s]
Downloading land_sea_mask.npy: 100%|██████████| 192k/192k [00:00<00:00, 84.8MB/s]
Downloading topography.npy: 0%| | 0.00/192k [00:00<?, ?B/s]
Downloading topography.npy: 100%|██████████| 192k/192k [00:00<00:00, 23.2MB/s]
DLESyM LatLon input coord shapes: [('batch', (0,)), ('time', (0,)), ('lead_time', (9,)), ('variable', (11,)), ('lat', (721,)), ('lon', (1440,))]
DLESyM HPX input coord shapes: [('batch', (0,)), ('time', (0,)), ('lead_time', (9,)), ('variable', (9,)), ('face', (12,)), ('height', (64,)), ('width', (64,))]
Lat-lon input variable names: ['z500' 'z1000' 't2m' 'tcwv' 't850' 'z250' 'sst' 'u10m' 'v10m' 'z300'
'z700']
Lat-lon output variable names: ['z500' 'tau300-700' 'z1000' 't2m' 'tcwv' 't850' 'z250' 'ws10m' 'sst']
HEALPix input variable names: ['z500' 'tau300-700' 'z1000' 't2m' 'tcwv' 't850' 'z250' 'ws10m' 'sst']
HEALPix output variable names: ['z500' 'tau300-700' 'z1000' 't2m' 'tcwv' 't850' 'z250' 'ws10m' 'sst']
Making Predictions, Regridding, and Selecting Outputs#
Let’s now pull some example data and make predictions with the model. As the
data source provides lat/lon data, we can use the earth2studio.models.px.DLESyMLatLon
model.
In addition, we demonstrate how to use the regridding utilities provided by
DLESyMLatLon to regrid onto the HEALPix grid. The earth2studio.models.px.DLESyM
model can then be used directly with the HEALPix data.
Finally, a key aspect of the DLESyM model is that it makes predictions for the atmosphere and ocean components at different timesteps, because the atmosphere is faster-evolving than the ocean. The atmosphere is predicted every 6 hours, while the ocean is only predicted every 48 hours. Thus, not all output lead times are valid for the ocean component. For convenience, we can use a method that selects only the valid outputs for each of the atmosphere and ocean components.
ic_date = np.datetime64("2021-06-15")
# Fetch some example data
x, coords = fetch_data(
source=data,
time=np.array([ic_date]),
variable=np.array(in_coords_ll["variable"]),
lead_time=in_coords_ll["lead_time"],
device=device,
)
# Can call the `DLESyMLatLon` model directly with the input lat/lon data
y, y_coords = model_ll(x, coords)
# Or, we can use the pre-processing and regridding utilities to regrid the data onto
# the HEALPix grid, and then run directly with `DLESyM`, which expects HEALPix data
x_prep, coords_prep = model_ll._prepare_derived_variables(x, coords)
x_hpx, coords_hpx = model_ll.to_hpx(x_prep), model_ll.coords_to_hpx(coords_prep)
y_hpx, y_coords_hpx = model_hpx(x_hpx, coords_hpx)
# Retrieve the valid outputs for atmos/ocean components from the predictions
y_atmos, y_atmos_coords = model_ll.retrieve_valid_atmos_outputs(y, y_coords)
y_ocean, y_ocean_coords = model_ll.retrieve_valid_ocean_outputs(y, y_coords)
print(
"Atmosphere outputs (variables, lead_time [hrs]):",
y_atmos_coords["variable"],
y_atmos_coords["lead_time"].astype("timedelta64[h]"),
)
print(
"Ocean outputs (variables, lead_time [hrs]):",
y_ocean_coords["variable"],
y_ocean_coords["lead_time"].astype("timedelta64[h]"),
)
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.188 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.189 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.190 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.191 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.192 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.193 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.194 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.195 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.196 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.197 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.198 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.278 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064592.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.316 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064592.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.327 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064592.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.331 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064592.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.333 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064592.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.334 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064592.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.336 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064592.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.337 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064592.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.343 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064592.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.372 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064592.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:12.395 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064592.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:06, 1.53it/s]
Fetching ARCO data: 36%|███▋ | 4/11 [00:00<00:01, 6.30it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:02<00:02, 1.90it/s]
Fetching ARCO data: 91%|█████████ | 10/11 [00:02<00:00, 3.93it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:02<00:00, 3.72it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.147 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.148 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.148 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.149 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.150 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.150 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.150 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.151 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.151 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.152 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.152 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.226 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064598.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.227 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064598.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.227 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064598.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.228 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064598.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.229 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064598.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.229 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064598.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.233 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064598.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.235 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064598.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.239 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064598.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.272 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064598.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:15.291 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064598.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.59it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:01, 5.83it/s]
Fetching ARCO data: 36%|███▋ | 4/11 [00:00<00:00, 7.21it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:01<00:02, 2.50it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:02<00:01, 2.92it/s]
Fetching ARCO data: 73%|███████▎ | 8/11 [00:02<00:01, 2.97it/s]
Fetching ARCO data: 82%|████████▏ | 9/11 [00:02<00:00, 3.64it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:02<00:00, 4.14it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.810 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.811 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.811 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.812 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.812 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.813 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.813 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.814 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.815 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.815 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.816 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.891 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064604.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.892 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064604.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.893 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064604.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.895 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064604.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.897 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064604.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.900 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064604.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.901 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064604.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.960 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064604.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.963 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064604.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:17.964 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064604.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.98it/s]
2025-05-16 00:40:18.035 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064604.0.0 to local cache
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.98it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:01, 5.52it/s]
Fetching ARCO data: 27%|██▋ | 3/11 [00:00<00:01, 4.84it/s]
Fetching ARCO data: 36%|███▋ | 4/11 [00:00<00:01, 5.00it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:01<00:01, 2.85it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:02<00:02, 1.70it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:03<00:00, 4.05it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:03<00:00, 3.55it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.910 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.911 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.912 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.912 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.913 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.913 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.914 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.915 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.915 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.916 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.916 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.987 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064610.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.988 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064610.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.989 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064610.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.989 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064610.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.991 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064610.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.993 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064610.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.994 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064610.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.994 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064610.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:20.995 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064610.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:21.000 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064610.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:21.014 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064610.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.40it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:02, 4.04it/s]
Fetching ARCO data: 27%|██▋ | 3/11 [00:00<00:02, 3.86it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:02<00:02, 2.11it/s]
Fetching ARCO data: 91%|█████████ | 10/11 [00:02<00:00, 4.08it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:02<00:00, 3.86it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.764 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.765 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.766 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.766 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.767 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.767 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.768 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.768 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.769 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.769 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.770 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.839 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064616.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.841 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064616.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.842 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064616.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.842 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064616.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.847 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064616.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.848 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064616.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.850 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064616.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.851 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064616.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.866 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064616.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.908 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064616.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:23.911 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064616.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.28it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:02, 3.00it/s]
Fetching ARCO data: 45%|████▌ | 5/11 [00:00<00:00, 7.37it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:01<00:01, 2.65it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:03<00:02, 1.62it/s]
Fetching ARCO data: 91%|█████████ | 10/11 [00:03<00:00, 3.20it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:03<00:00, 3.34it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.063 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.064 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.064 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.065 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.065 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.066 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.066 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.067 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.067 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.068 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.068 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.140 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064622.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.141 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064622.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.144 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064622.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.145 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064622.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.146 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064622.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.148 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064622.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.153 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064622.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.205 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064622.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.206 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064622.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:27.211 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064622.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.51it/s]
2025-05-16 00:40:27.392 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064622.0.0 to local cache
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.51it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:02, 4.40it/s]
Fetching ARCO data: 27%|██▋ | 3/11 [00:00<00:01, 5.19it/s]
Fetching ARCO data: 36%|███▋ | 4/11 [00:00<00:01, 5.23it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:02<00:02, 2.38it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:02<00:01, 2.01it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:03<00:00, 3.96it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:03<00:00, 3.51it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.197 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.198 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.198 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.199 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.199 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.200 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.200 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.201 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.201 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.201 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.202 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.273 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064628.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.275 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064628.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.275 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064628.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.276 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064628.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.277 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064628.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.277 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064628.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.278 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064628.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.280 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064628.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.284 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064628.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:30.288 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064628.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.85it/s]
2025-05-16 00:40:30.466 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064628.0.0.0 to local cache
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.85it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:02, 3.25it/s]
Fetching ARCO data: 36%|███▋ | 4/11 [00:00<00:01, 5.43it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:01<00:01, 3.58it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:02<00:01, 2.66it/s]
Fetching ARCO data: 73%|███████▎ | 8/11 [00:02<00:01, 2.54it/s]
Fetching ARCO data: 82%|████████▏ | 9/11 [00:02<00:00, 3.17it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:02<00:00, 3.88it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.034 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.034 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.035 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.035 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.036 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.036 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.037 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.037 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.038 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.038 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.039 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.109 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064634.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.112 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064634.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.113 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064634.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.114 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064634.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.114 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064634.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.115 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064634.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.116 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064634.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.116 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064634.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:33.176 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064634.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.00it/s]
2025-05-16 00:40:33.285 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064634.0.0.0 to local cache
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.00it/s]
2025-05-16 00:40:33.300 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064634.0.0 to local cache
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.00it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:01, 5.43it/s]
Fetching ARCO data: 27%|██▋ | 3/11 [00:00<00:01, 5.93it/s]
Fetching ARCO data: 45%|████▌ | 5/11 [00:00<00:01, 5.03it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:01<00:02, 2.46it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:02<00:01, 2.14it/s]
Fetching ARCO data: 91%|█████████ | 10/11 [00:02<00:00, 4.13it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:02<00:00, 3.96it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.818 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.819 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.820 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.820 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.821 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.821 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.822 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.822 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.823 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.823 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.824 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.898 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_u_component_of_wind/1064640.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.899 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064640.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.899 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/total_column_water_vapour/1064640.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.901 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/10m_v_component_of_wind/1064640.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.901 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/temperature/1064640.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.902 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/sea_surface_temperature/1064640.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.902 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/2m_temperature/1064640.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.956 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064640.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.958 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064640.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:35.964 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064640.0.0.0 to local cache
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.36it/s]
2025-05-16 00:40:36.041 | DEBUG | earth2studio.data.utils:_make_local_details:629 - Copying /gcp-public-data-arco-era5/ar/full_37-1h-0p25deg-chunk-1.zarr-v3/geopotential/1064640.0.0.0 to local cache
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:01, 5.36it/s]
Fetching ARCO data: 18%|█▊ | 2/11 [00:00<00:01, 5.32it/s]
Fetching ARCO data: 45%|████▌ | 5/11 [00:00<00:00, 6.86it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:01<00:02, 2.48it/s]
Fetching ARCO data: 64%|██████▎ | 7/11 [00:02<00:01, 2.01it/s]
Fetching ARCO data: 73%|███████▎ | 8/11 [00:02<00:01, 2.41it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:02<00:00, 3.74it/s]
Atmosphere outputs (variables, lead_time [hrs]): ['z500' 'tau300-700' 'z1000' 't2m' 'tcwv' 't850' 'z250' 'ws10m'] [ 6 12 18 24 30 36 42 48 54 60 66 72 78 84 90 96]
Ocean outputs (variables, lead_time [hrs]): ['sst'] [48 96]
Model Iteration for Longer Forecasts#
Similar to other models in earth2studio, we can use the model iterator to loop over forecasted outputs. A single step of the DLESyM model produces predictions over 4 days (96 hours), so to make a sub-seasonal forecast we can take 15 steps for a total of 60 days.
n_steps = 16
model_iter_ll = model_ll.create_iterator(x, coords)
for i in range(n_steps):
x, x_coords = next(model_iter_ll)
if i > 0: # Don't retrieve the first step as it is the initial condition
x_atmos, x_atmos_coords = model_ll.retrieve_valid_atmos_outputs(x, x_coords)
x_ocean, x_ocean_coords = model_ll.retrieve_valid_ocean_outputs(x, x_coords)
print(f"Completed forecast with {n_steps} steps")
Completed forecast with 16 steps
Using Built-in Deterministic Workflow#
Because the DLESyMLatLon model permits usage of data coming directly from an earth2studio data source, we can use the built-in deterministic workflow to generate a forecast as well. The only caveat is we need to explitcitly specify the output lead time coordinates that will be generated by the model, since it has different input and output lead time dimensions. %%
import earth2studio.run as run
from earth2studio.io import KVBackend
io = KVBackend()
output_coords = model_ll.output_coords(coords)
inp_lead_time = model_ll.input_coords()["lead_time"]
out_lead_times = [
output_coords["lead_time"] + output_coords["lead_time"][-1] * i
for i in range(n_steps)
]
output_coords["lead_time"] = np.concatenate([inp_lead_time, *out_lead_times])
io = run.deterministic(
[ic_date], n_steps, model_ll, data, io, output_coords=output_coords
)
ds = io.to_xarray()
print(ds)
2025-05-16 00:40:47.449 | INFO | earth2studio.run:deterministic:75 - Running simple workflow!
2025-05-16 00:40:47.449 | INFO | earth2studio.run:deterministic:82 - Inference device: cuda
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.453 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.454 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.455 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.455 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.456 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.456 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.457 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.457 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.458 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.458 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.459 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.59it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 21.63it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 25.66it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.886 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.887 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.887 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.888 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.888 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.889 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.889 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.890 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.890 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.891 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:47.891 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 3.73it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 18.62it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 23.66it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.355 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.355 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.356 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.356 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.357 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.357 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.358 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.358 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.359 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.359 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.360 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.03it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 17.92it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 24.01it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.817 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.818 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.818 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.819 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.819 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.820 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.820 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.821 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.821 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.822 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:48.822 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-13T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 3.91it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 18.83it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 23.81it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.283 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.284 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.284 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.285 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.285 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.286 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.286 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.287 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.287 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.288 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.288 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.04it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 17.87it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 24.28it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.740 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.741 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.741 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.742 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.743 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.743 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.744 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.744 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.745 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.745 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:49.746 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T06:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.17it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 20.61it/s]
Fetching ARCO data: 82%|████████▏ | 9/11 [00:00<00:00, 23.31it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 24.17it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.199 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.200 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.200 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.201 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.201 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.202 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.202 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.203 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.203 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.204 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.204 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T12:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.13it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 21.09it/s]
Fetching ARCO data: 91%|█████████ | 10/11 [00:00<00:00, 27.30it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 24.52it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.652 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.653 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.653 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.654 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.654 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.655 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.655 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.656 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.656 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.657 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:50.657 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-14T18:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.15it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 17.12it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 24.32it/s]
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.108 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t2m at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.109 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: t850 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.110 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z500 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.110 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: sst at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.111 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: v10m at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.111 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: tcwv at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.112 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z700 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.112 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z1000 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.113 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z250 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.113 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: z300 at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
2025-05-16 00:40:51.114 | DEBUG | earth2studio.data.arco:fetch_array:252 - Fetching ARCO zarr array for variable: u10m at 2021-06-15T00:00:00
Fetching ARCO data: 0%| | 0/11 [00:00<?, ?it/s]
Fetching ARCO data: 9%|▉ | 1/11 [00:00<00:02, 4.21it/s]
Fetching ARCO data: 55%|█████▍ | 6/11 [00:00<00:00, 18.24it/s]
Fetching ARCO data: 100%|██████████| 11/11 [00:00<00:00, 24.76it/s]
2025-05-16 00:40:51.777 | SUCCESS | earth2studio.run:deterministic:106 - Fetched data from ARCO
2025-05-16 00:40:52.532 | INFO | earth2studio.run:deterministic:136 - Inference starting!
Running inference: 0%| | 0/17 [00:00<?, ?it/s]
Running inference: 6%|▌ | 1/17 [00:00<00:08, 1.86it/s]
Running inference: 12%|█▏ | 2/17 [00:11<01:39, 6.65s/it]
Running inference: 18%|█▊ | 3/17 [00:22<02:00, 8.62s/it]
Running inference: 24%|██▎ | 4/17 [00:33<02:04, 9.57s/it]
Running inference: 29%|██▉ | 5/17 [00:44<02:00, 10.06s/it]
Running inference: 35%|███▌ | 6/17 [00:55<01:54, 10.37s/it]
Running inference: 41%|████ | 7/17 [01:06<01:45, 10.57s/it]
Running inference: 47%|████▋ | 8/17 [01:17<01:36, 10.67s/it]
Running inference: 53%|█████▎ | 9/17 [01:28<01:26, 10.77s/it]
Running inference: 59%|█████▉ | 10/17 [01:39<01:15, 10.85s/it]
Running inference: 65%|██████▍ | 11/17 [01:50<01:05, 10.93s/it]
Running inference: 71%|███████ | 12/17 [02:01<00:54, 10.95s/it]
Running inference: 76%|███████▋ | 13/17 [02:12<00:43, 10.95s/it]
Running inference: 82%|████████▏ | 14/17 [02:23<00:32, 10.97s/it]
Running inference: 88%|████████▊ | 15/17 [02:34<00:21, 10.98s/it]
Running inference: 94%|█████████▍| 16/17 [02:45<00:10, 11.00s/it]
Running inference: 100%|██████████| 17/17 [02:56<00:00, 10.99s/it]
Running inference: 100%|██████████| 17/17 [02:56<00:00, 10.37s/it]
2025-05-16 00:43:48.844 | SUCCESS | earth2studio.run:deterministic:146 - Inference complete
<xarray.Dataset> Size: 10GB
Dimensions: (time: 1, lead_time: 265, lat: 721, lon: 1440)
Coordinates:
* time (time) datetime64[s] 8B 2021-06-15
* lead_time (lead_time) timedelta64[ns] 2kB -2 days +00:00:00 ... 64 days...
* lat (lat) float64 6kB 90.0 89.75 89.5 89.25 ... -89.5 -89.75 -90.0
* lon (lon) float64 12kB 0.0 0.25 0.5 0.75 ... 359.0 359.2 359.5 359.8
Data variables:
z500 (time, lead_time, lat, lon) float32 1GB 5.253e+04 ... 4.724e+04
tau300-700 (time, lead_time, lat, lon) float32 1GB 5.85e+04 ... 5.453e+04
z1000 (time, lead_time, lat, lon) float32 1GB 890.4 890.4 ... -936.4
t2m (time, lead_time, lat, lon) float32 1GB 273.7 273.7 ... 214.5
tcwv (time, lead_time, lat, lon) float32 1GB 8.898 8.898 ... 0.3016
t850 (time, lead_time, lat, lon) float32 1GB 265.6 265.6 ... 235.4
z250 (time, lead_time, lat, lon) float32 1GB 9.844e+04 ... 8.992e+04
ws10m (time, lead_time, lat, lon) float32 1GB 0.0292 0.0292 ... 6.945
sst (time, lead_time, lat, lon) float32 1GB 271.5 271.5 ... 272.1
Plotting the Outputs#
Let’s plot some of the forecasted outputs for the atmosphere and ocean components.
import cartopy.crs as ccrs
import cartopy.feature as cfeature
import matplotlib.pyplot as plt
# lat = x_atmos_coords["lat"]
# lon = x_atmos_coords["lon"]
atmos_var, atmos_units = "ws10m", "m/s"
ocean_var, ocean_units = "sst", "K"
# atmos_var_idx = list(x_atmos_coords["variable"]).index(atmos_var)
# ocean_var_idx = list(x_ocean_coords["variable"]).index(ocean_var)
lead_time = ds.lead_time.values[-1]
plt.close("all")
# Create a Robinson projection
projection = ccrs.Robinson()
# Create a figure and axes with the specified projection
fig, axs = plt.subplots(1, 2, subplot_kw={"projection": projection}, figsize=(15, 6))
# Plot the field using pcolormesh
im = axs[0].pcolormesh(
ds.lon.values,
ds.lat.values,
ds[atmos_var].sel(time=ic_date, lead_time=lead_time).values,
transform=ccrs.PlateCarree(),
cmap="cividis",
)
# Set title
axs[0].set_title(
f"Initialization: {ic_date} - Lead time: {lead_time.astype('timedelta64[h]')}"
)
# Add coastlines and gridlines
axs[0].coastlines()
axs[0].gridlines()
cbar = fig.colorbar(im, ax=axs[0], orientation="horizontal", pad=0.05)
cbar.set_label(f"{atmos_var} [{atmos_units}]")
# Plot the ocean component
im = axs[1].pcolormesh(
ds.lon.values,
ds.lat.values,
ds[ocean_var].sel(time=ic_date, lead_time=lead_time).values,
transform=ccrs.PlateCarree(),
cmap="Spectral_r",
)
axs[1].set_title(
f"Initialization: {ic_date} - Lead time: {lead_time.astype('timedelta64[h]')}"
)
# Add coastlines and gridlines
axs[1].add_feature(cfeature.LAND, color="grey", zorder=100)
axs[1].coastlines()
axs[1].gridlines()
cbar = fig.colorbar(im, ax=axs[1], orientation="horizontal", pad=0.05)
cbar.set_label(f"{ocean_var} [{ocean_units}]")
plt.tight_layout()
plt.savefig("outputs/14_ws10m_sst_prediction.png")
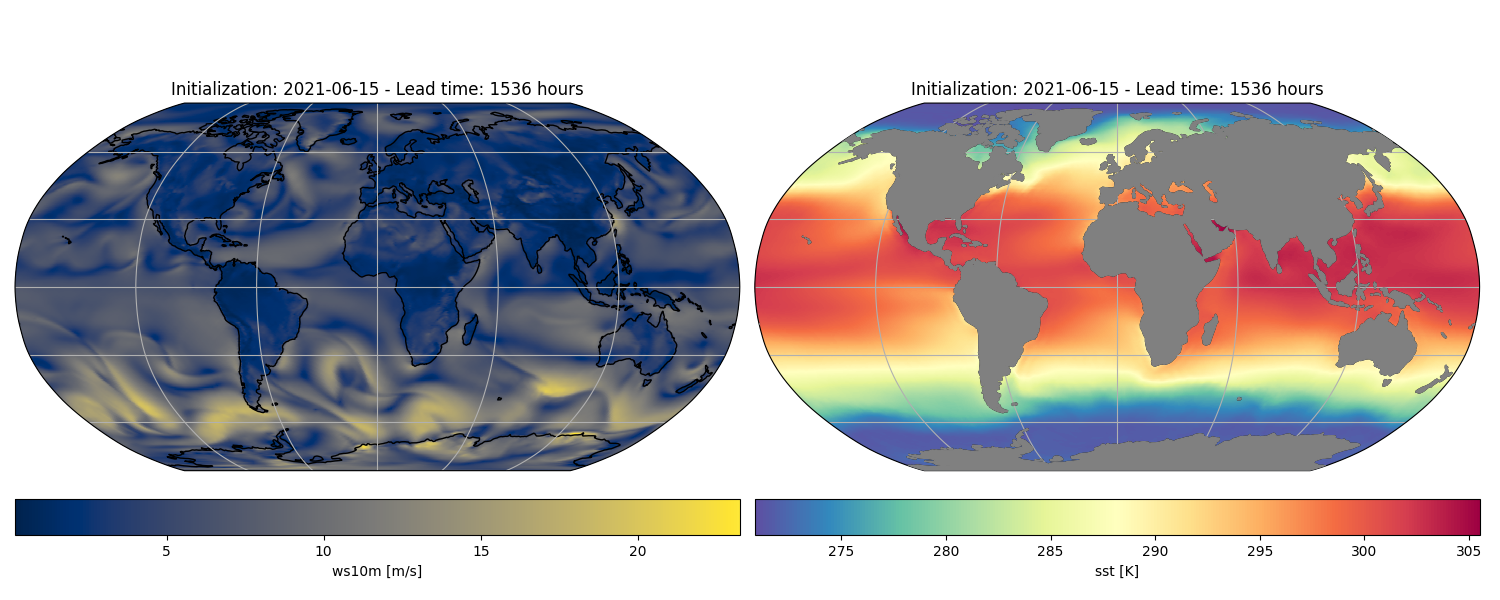
Total running time of the script: (5 minutes 55.198 seconds)