Molecular docking via DC-QAOA¶
Drugs often work by binding to an active site of a protein, inhibiting or activating its function for some therapeutic purpose. Finding new candidate drugs is extremely difficult. The study of molecular docking helps guide this search and involves the prediction of how strongly a certain ligand (drug) will bind to its target (usually a protein).
One of the primary challenges to molecular docking arises from the many geometric degrees of freedom present in proteins and ligands, making it difficult to predict the optimal orientation and assess if the drug is a good candidate or not. One solution is to formulate the problem as a mathematical optimization problem where the optimal solution corresponds to the most likely ligand-protein configuration. This optimization problem can be solved on a quantum computer using methods like the Quantum Approximate Optimization Algorithm (QAOA). This tutorial demonstrates how this paper used digitized-counteradiabatic (DC) QAOA to study molecular docking. This tutorial assumes you have an understanding of QAOA, if not, please see the CUDA-Q MaxCut tutorial found here.
The next section provides more detail on the problem setup followed by CUDA-Q implementations below.
Setting up the Molecular Docking Problem¶
The figure from the paper provides a helpful diagram for understanding the workflow.
There are 6 key steps: 1. The experimental protein and ligand structures are determined and used to select pharmacores, or an important chemical group that will govern the chemical interactions. 2. Two labeled distance graphs (LAGs) of size \(N\) and \(M\) represent the protein and the ligand, respectively. Each node corresponds to a pharmacore and each edge weight corresponds to the distance between pharmacores. 3. A \(M*N\) node binding interaction graph (BIG) is created from the LAGs. Each node in the BIG graph corresponds to a pair of pharmacores, one from the ligand and the other from the protein. The existence of edges between nodes in the BIG graph are determined from the LAGs and correspond to interactions that can feesibly coexist. Therefore, cliques in the graph correspond to mutually possible interactions. 4. The problem is mapped to a QAOA circuit and corresponding Hamiltonian. From there, the ground state solution is determined. 5. The ground state will produce the maximum weighted clique which corresponds to the best (most strongly bound) orientation of the ligand and protein. 6. The predicted docking structure is interpreted from the QAOA result and is used for further analysis.
CUDA-Q Implementation¶
First, the appropriate libraries are imported and the nvidia
backend is selected to run on GPUs if available.
[6]:
import cudaq
from cudaq import spin
import numpy as np
The block below defines two of the BIG data sets from the paper. The first is a smaller example, but it can be swapped with the commented out example below at your discretion. The weights are specified for each node based on the nature of the ligand and protein pharmacores represented by the node.
[7]:
# The two graph inputs from the paper
# BIG 1
nodes = [0, 1, 2, 3, 4, 5]
qubit_num = len(nodes)
edges = [[0, 1], [0, 2], [0, 4], [0, 5], [1, 2], [1, 3], [1, 5], [2, 3], [2, 4],
[3, 4], [3, 5], [4, 5]]
non_edges = [
[u, v] for u in nodes for v in nodes if u < v and [u, v] not in edges
]
print('Edges: ', edges)
print('Non-Edges: ', non_edges)
weights = [0.6686, 0.6686, 0.6686, 0.1453, 0.1453, 0.1453]
penalty = 6.0
num_layers = 3
# BIG 2 (More expensive simulation)
#nodes=[0,1,2,3,4,5,6,7]
#qubit_num=len(nodes)
#edges=[[0,1],[0,2],[0,5],[0,6],[0,7],[1,2],[1,4],[1,6],[1,7],[2,4],[2,5],[2,7],[3,4],[3,5],[3,6],\
# [4,5],[4,6],[5,6]]
#non_edges=[[u,v] for u in nodes for v in nodes if u<v and [u,v] not in edges]
#print('Edges: ', edges)
#print('Non-edges: ', non_edges)
#weights=[0.6686,0.6686,0.6886,0.1091,0.0770,0.0770,0.0770,0.0770]
#penalty=8.0
#num_layers=8
Edges: [[0, 1], [0, 2], [0, 4], [0, 5], [1, 2], [1, 3], [1, 5], [2, 3], [2, 4], [3, 4], [3, 5], [4, 5]]
Non-Edges: [[0, 3], [1, 4], [2, 5]]
Next, the Hamiltonian is constructed:
The first term concerns the vertices and the weights of the given pharmacores. The second term is a penalty term that penalizes edges of the graph with no interactions. The penalty \(P\) is set by the user and is defined as 6 in the cell above. The function below returns the Hamiltonian as a CUDA-Q spin_op
object.
[8]:
# Generate the Hamiltonian
def ham_clique(penalty, nodes, weights, non_edges) -> cudaq.SpinOperator:
spin_ham = 0
for wt, node in zip(weights, nodes):
#print(wt,node)
spin_ham += 0.5 * wt * spin.z(node)
spin_ham -= 0.5 * wt * spin.i(node)
for non_edge in non_edges:
u, v = (non_edge[0], non_edge[1])
#print(u,v)
spin_ham += penalty / 4.0 * (spin.z(u) * spin.z(v) - spin.z(u) -
spin.z(v) + spin.i(u) * spin.i(v))
return spin_ham
The code below strips the Hamiltonian into a list of coefficients and corresponding Pauli words which can be passed into a quantum kernel.
[9]:
# Collect coefficients from a spin operator so we can pass them to a kernel
def term_coefficients(ham: cudaq.SpinOperator) -> list[complex]:
result = []
for term in ham:
result.append(term.evaluate_coefficient())
return result
# Collect Pauli words from a spin operator so we can pass them to a kernel
def term_words(ham: cudaq.SpinOperator) -> list[str]:
# Our kernel uses these words to apply exp_pauli to the entire state.
# we hence ensure that each pauli word covers the entire space.
result = []
for term in ham:
result.append(term.get_pauli_word(qubit_num))
return result
ham = ham_clique(penalty, nodes, weights, non_edges)
print(ham)
coef = term_coefficients(ham)
words = term_words(ham)
print(term_coefficients(ham))
print(term_words(ham))
(0+0i) + (-1.1657+0i) * Z0 + (-0.3343+0i) * I0 + (-1.1657+0i) * Z1 + (-0.3343+0i) * I1 + (-1.1657+0i) * Z2 + (-0.3343+0i) * I2 + (-1.42735+0i) * Z3 + (-0.07265+0i) * I3 + (-1.42735+0i) * Z4 + (-0.07265+0i) * I4 + (-1.42735+0i) * Z5 + (-0.07265+0i) * I5 + (1.5+0i) * Z0Z3 + (1.5+0i) * I0I3 + (1.5+0i) * Z1Z4 + (1.5+0i) * I1I4 + (1.5+0i) * Z2Z5 + (1.5+0i) * I2I5
[0j, (-1.1657+0j), (-0.3343-0j), (-1.1657+0j), (-0.3343-0j), (-1.1657+0j), (-0.3343-0j), (-1.42735+0j), (-0.07265-0j), (-1.42735+0j), (-0.07265-0j), (-1.42735+0j), (-0.07265-0j), (1.5+0j), (1.5+0j), (1.5+0j), (1.5+0j), (1.5+0j), (1.5+0j)]
['IIIIII', 'ZIIIII', 'IIIIII', 'IZIIII', 'IIIIII', 'IIZIII', 'IIIIII', 'IIIZII', 'IIIIII', 'IIIIZI', 'IIIIII', 'IIIIIZ', 'IIIIII', 'ZIIZII', 'IIIIII', 'IZIIZI', 'IIIIII', 'IIZIIZ', 'IIIIII']
The kernel below defines a DC-QAOA circuit. What makes the approach “DC” is the inclusion of additional counteradiabatic terms to better drive the optimization to the ground state. These terms are digitized and applied as additional operations following each QAOA layer. The increase in parameters is hopefully offset by requiring fewer layers. In this example, the DC terms are the additional parameterized \(Y\) operations applied to each qubit. These can be commented out to run conventional QAOA.
[10]:
@cudaq.kernel
def dc_qaoa(qubit_num:int, num_layers:int, thetas:list[float],\
coef:list[complex], words:list[cudaq.pauli_word]):
qubits = cudaq.qvector(qubit_num)
h(qubits)
count = 0
for p in range(num_layers):
for i in range(len(coef)):
exp_pauli(thetas[count] * coef[i].real, qubits, words[i])
count += 1
for j in range(qubit_num):
rx(thetas[count], qubits[j])
count += 1
#Comment out this for loop for conventional QAOA
for k in range(qubit_num):
ry(thetas[count], qubits[k])
count += 1
The classical optimizer for the QAOA procedure can be specified as one of the built-in CUDA-Q optimizers, in this case Nelder Mead. The parameter count is defined for DC-QAOA, but can be swapped with the commented line below for conventional QAOA.
[11]:
# Specify the optimizer and its initial parameters.
optimizer = cudaq.optimizers.NelderMead()
#Specify random seeds
np.random.seed(13)
cudaq.set_random_seed(13)
# if dc_qaoa used
parameter_count = (2 * qubit_num + len(coef)) * num_layers
# if qaoa used
# parameter_count=(qubit_num+len(coef))*num_layers
print('Total number of parameters: ', parameter_count)
optimizer.initial_parameters = np.random.uniform(-np.pi / 8, np.pi / 8,
parameter_count)
print("Initial parameters = ", optimizer.initial_parameters)
Total number of parameters: 93
Initial parameters = [0.21810696323572243, -0.20613464375211488, 0.2546877639814583, 0.3657985647468064, 0.37118004688049144, -0.03656087558321203, 0.08564174998504231, 0.21639801853794682, 0.11122286088634259, 0.1743727097033635, -0.36518146001762486, -0.15829741539542244, -0.3467434780387345, 0.28043500852894776, -0.09986021299050934, 0.14125225086023052, -0.19141728018199775, -0.11970943368650361, -0.3853063093646483, -0.1112643868789806, 0.3527177454825464, -0.22156160012057186, -0.1418496891385843, 0.32811766468303116, -0.367642000671186, -0.34158180583996006, 0.10196745745501312, 0.29359239180502594, -0.3858537615546677, 0.19366130907065582, 0.24570488114056754, -0.3332307385378807, 0.12287973244618389, 0.007274514934614895, -0.015799547372526146, 0.3578070967202224, -0.39268963055535144, -0.19872246354138554, 0.16668715544467982, -0.13777293592446055, -0.17514665212709513, 0.15350249947988204, 0.32872977428061945, -0.20068831419712105, -0.032919322131134854, -0.19399909325771983, -0.09477141125241506, 0.08210460401106645, 0.21392577760158515, -0.3393568044538389, 0.14615087942938465, 0.03790339186006314, -0.2843250892879255, -0.3151384847055956, -0.19983741137121905, -0.27348611567665115, 0.33457528180906904, 0.14145414847455462, -0.20604220093940323, 0.05410235084309195, 0.04447870918600966, -0.3355714098595045, 0.266806440171265, -0.07436189654442632, -0.2789176729721685, -0.2427508182662484, -0.007351219158674538, 0.16652355413124942, 0.3808697740391629, 0.2943566301288585, -0.0007526597041920824, -0.3088342705284747, 0.3245365797019699, -0.10609475622422088, -0.21473737933378598, 0.29250730067679076, -0.28560348266311547, -0.20704653805110443, 0.07492639208926116, 0.050204699769995864, 0.3604464956189818, -0.036725741805424705, -0.2914156467694673, 0.20464937477384804, -0.2343360494257549, -0.2546811698770065, -0.04938739737483455, -0.1254588720659109, 0.3669797333052197, -0.2803666634362036, 0.27061563884884454, 0.13305127120906368, -0.3068512076427221]
A cost function is specified which computes the expectation value of the DC-QAOA circuit and the Hamiltonian using the observe
function. Running the optimization returns the minimized expectation value and the optimal parameters.
[12]:
cost_values = []
def objective(parameters):
cost = cudaq.observe(dc_qaoa, ham, qubit_num, num_layers, parameters, coef,
words).expectation()
cost_values.append(cost)
return cost
# Optimize!
optimal_expectation, optimal_parameters = optimizer.optimize(
dimensions=parameter_count, function=objective)
print('optimal_expectation =', optimal_expectation)
print('optimal_parameters =', optimal_parameters)
optimal_expectation = -2.005710024421982
optimal_parameters = [1.8481888913540985, -0.2535399481030596, 0.22783474501904072, 2.081416556947703, 0.3374107641822874, -0.05224802127495901, 1.8679499667316164, 2.009143330388614, 0.1339973108317789, 0.2652751145535136, 1.324101519994548, 1.5559093725862327, -0.16508452001869578, 0.10055168392123187, 1.4335293611790534, -0.022802387382032274, -0.02345217217095934, 0.025049619373114198, -0.32382028011884517, 1.1592457772894376, 0.5114936288385474, -0.14306579846281064, -0.32504364746711095, 1.5987201329360095, -0.47283036988738203, -0.2879606126466135, 0.03195039154942317, 1.6485828925522248, -0.8013579153446403, -0.025680220483288878, 0.37302396767692614, 1.5188370864376863, 2.106660523866304, -0.09725921137896973, 0.17491777895553523, 0.5884424387073575, 1.0185444633156848, 1.2506750467643695, 1.5730001880356774, 0.923145372786352, -0.3016838127319703, 0.10130783752008649, 0.34049803711462423, -0.2742308863536732, -0.0843331582214344, -0.304728036625607, 0.03164048010752481, -0.16945553246060785, 0.21199415583984976, -0.333776464782457, 0.6762454214008358, 0.7077770344190998, -0.04315001481827706, -0.8685775873913754, -0.31256033232512964, -0.13726466931210604, -0.18103220051469332, -0.07014233979739845, -0.04255058220153306, 0.5213395683000333, -0.2522616199668185, -0.5922668326436884, 1.7533503557489256, -0.4243163991330685, 1.8047318156160967, -0.11962817999838339, 1.8264810507160347, 1.9427616058014427, 0.7014295339746117, 0.20606112998073237, 0.5143352145327803, -0.34597927642063775, 0.2594379052182792, -0.05203689954085833, -0.5794522822029166, 0.4906495726071997, -0.034324583542975434, -0.14861293759475658, -0.12344152798253367, 0.025496591883733336, 1.284665133192642, -0.3804169340191194, 0.24490229273798853, 0.17606447338069542, -0.3615511014437435, -0.32667907511589545, -0.02539630711633685, -0.6837298769178322, 0.5233213940389638, -0.10356768274588322, 0.3255850390639937, 0.23127621992973585, -0.44519166728496085]
Sampling the circuit with the optimal parameters allows for the most_probable
command to reveal the bitsting corresponding to the ideal graph partitioning solution. This indicates what sort of interactions are present in the ideal docking configuration
[13]:
shots = 200000
counts = cudaq.sample(dc_qaoa,
qubit_num,
num_layers,
optimal_parameters,
coef,
words,
shots_count=shots)
print(counts)
print('The MVWCP is given by the partition: ', counts.most_probable())
{ 011100:1 101010:6 110001:20 111000:199973 }
The MVWCP is given by the partition: 111000
The convergence of the optimization can be plotted below.
[14]:
import matplotlib.pyplot as plt
x_values = list(range(len(cost_values)))
y_values = cost_values
plt.plot(x_values, y_values)
plt.xlabel("Epochs")
plt.ylabel("Cost Value")
plt.show()
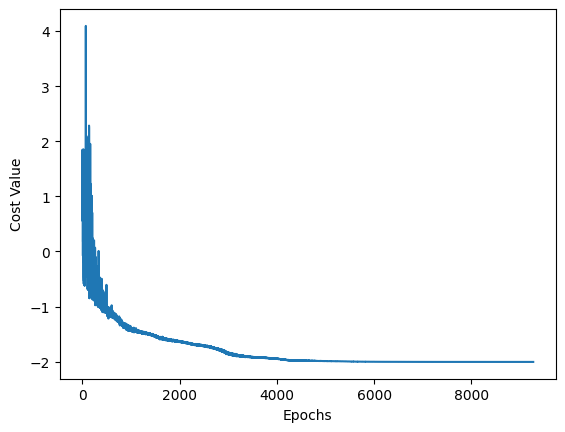