Quantum Hardware (QPU)¶
CUDA-Q supports submission to a set of hardware providers. To submit to a hardware backend, you need an account with the respective provider.
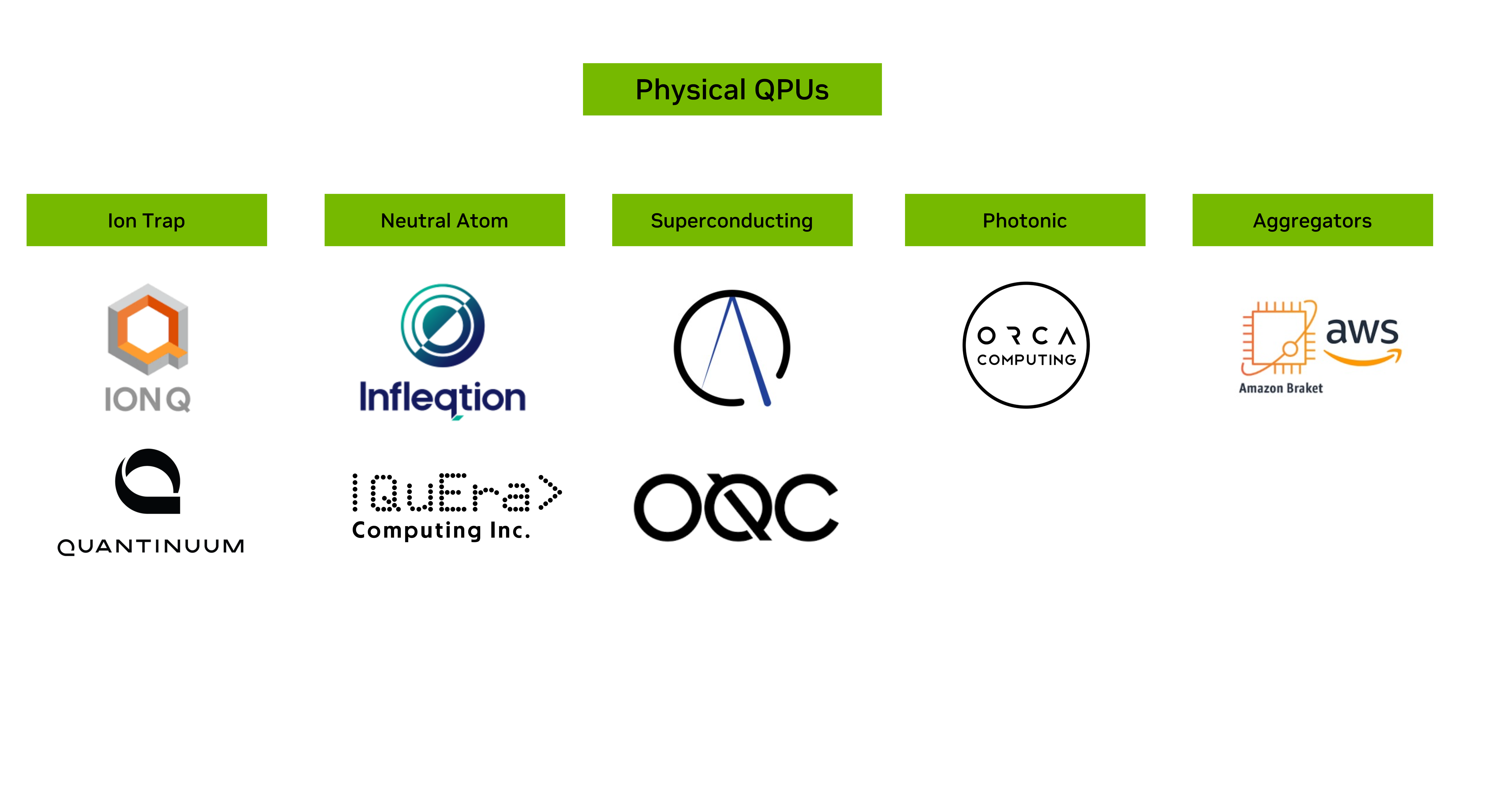
CUDA-Q supports submission to a set of hardware providers. To submit to a hardware backend, you need an account with the respective provider.